| public void load() { // String id = request.getParameter("id"); String actiontype = "save"; String style=request.getParameter("style"); if (id != null) { Xinxi xinxi = (Xinxi) DALBase.load("xinxi", "where id=" + id); if (xinxi != null) { request.setAttribute("xinxi", xinxi); } actiontype = "update"; } request.setAttribute("id", id); request.setAttribute("actiontype", actiontype); try { request.getRequestDispatcher("xinxiadd.jsp").forward(request,response); } catch (ServletException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } /****************************************************** *********************** 数据绑定内部支持********************* *******************************************************/ public void binding() { String filter = ""; // int pageindex = 1; int pagesize = 10; String title=request.getParameter("title"); String lanmuid=request.getParameter("lanmuid"); // 获取当前分页 if(title!=null) filter="where title like '%"+title+"%'"; else { filter=" where 1=1 "; } if(lanmuid!=null&&lanmuid!="") filter+=" and lanmuid="+lanmuid; System.out.println("filter="+filter); String currentpageindex = request.getParameter("currentpageindex"); // 当前页面尺寸 String currentpagesize = request.getParameter("pagesize"); // 设置当前页 if (currentpageindex != null) pageindex = new Integer(currentpageindex); // 设置当前页尺寸 if (currentpagesize != null) pagesize = new Integer(currentpagesize); List<Xinxi> listxinxi = DALBase.getPageEnity("xinxi", filter, pageindex, pagesize); int recordscount = DALBase.getRecordCount("xinxi", filter == null ? ": filter); request.setAttribute("listxinxi", listxinxi); PagerMetal pm = new PagerMetal(recordscount); // 设置尺寸 pm.setPagesize(pagesize); // 设置当前显示页 pm.setCurpageindex(pageindex); // 设置分页信息 request.setAttribute("pagermetal", pm); // 分发请求参数 dispatchParams(request, response); try { request.getRequestDispatcher("/admin/xinximanager.jsp").forward( request, response); } catch (ServletException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }解释每一行
时间: 2023-07-23 14:50:20 浏览: 53
这段代码是一个Java类中的两个方法,load()和binding()。
load()方法的作用是加载一个名为"xinxiadd.jsp"的页面,并在加载页面之前,根据请求参数中的id来判断是执行新增还是修改操作。如果id不为空,则表示执行修改操作,会从数据库中加载id对应的数据,并将数据设置为request的属性,然后设置请求参数actiontype为"update"。如果id为空,则表示执行新增操作,请求参数actiontype为"save"。最后使用request的getRequestDispatcher()方法来将请求转发到xinxiadd.jsp页面。
binding()方法的作用是进行数据绑定操作。首先获取请求参数title和lanmuid,根据这两个参数来组合过滤条件filter。然后获取请求参数currentpageindex和currentpagesize,来设置当前页和每页显示的数据条数。接着调用DALBase.getPageEnity()方法来获取符合过滤条件的数据列表listxinxi,并调用DALBase.getRecordCount()方法来获取总记录数recordscount。然后创建一个PagerMetal对象pm,用于设置分页信息,并将数据列表和分页信息设置为request的属性。最后使用getRequestDispatcher()方法将请求转发到admin/xinximanager.jsp页面。
相关问题
public void update() { String id = request.getParameter("id"); if (id == null) return; Xinxi xinxi = (Xinxi) DALBase.load(Xinxi.class, new Integer(id)); if (xinxi == null) return; String title = request.getParameter("title"); String pubren = request.getParameter("pubren"); String laiyuan=request.getParameter("laiyuan"); String clickcount = request.getParameter("clickcount"); String dcontent = request.getParameter("dcontent"); String tupian2 = request.getParameter("tupian2"); String lanmuid = request.getParameter("lanmuid"); String lanmuming = request.getParameter("lanmuming"); String tuijian=request.getParameter("tuijian"); String hot=request.getParameter("hot"); String zuixin=request.getParameter("zuixin"); String zhaiyao=request.getParameter("zhaiyao"); String style=request.getParameter("style"); SimpleDateFormat sdfxinxi = new SimpleDateFormat("yyyy-MM-dd"); xinxi.setZhaiyao(zhaiyao); xinxi.setTitle(title); xinxi.setPubren(pubren); xinxi.setLaiyuan(laiyuan); xinxi.setDcontent(dcontent); xinxi.setHot(hot!=null?1:0); xinxi.setTuijian(tuijian!=null?1:0); xinxi.setZuixin(zuixin!=null?1:0); xinxi.setTupian2(tupian2); xinxi.setLanmuid(new Integer(lanmuid)); xinxi.setLanmuming(lanmuming); DALBase.update(xinxi); //attachements(request, response, new Integer(xinxi.getId()).toString()); //binding(request, response); try { if(style!=null&&style.equals("admin")) response.sendRedirect("xinximanager.do?actiontype=get&seedid=201&lanmuid="+xinxi.getLanmuid()); if(style!=null&&style.equals("huiyuan")) response.sendRedirect("../e/myxinximanager.jsp?seedid=m2"); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } /****************************************************** *******************解释每一行代码
这是一个Java代码段,实现了一个名为"update()"的方法。这个方法通过获取HTTP请求中的参数,更新数据库中的一个名为"Xinxi"的实例对象。下面是每一行代码的解释:
1. public void update() {
定义了一个名为"update()"的公共方法,它没有任何参数,也没有返回值。
2. String id = request.getParameter("id");
获取HTTP请求中名为"id"的参数,并将其存储在一个名为"id"的字符串变量中。
3. if (id == null) return;
如果"id"为null,直接退出方法。
4. Xinxi xinxi = (Xinxi) DALBase.load(Xinxi.class, new Integer(id));
通过id参数,从数据库中加载一个名为"Xinxi"的对象实例,并将其存储在名为"xinxi"的变量中。
5. if (xinxi == null) return;
如果"xinxi"为null,直接退出方法。
6. String title = request.getParameter("title");
获取HTTP请求中名为"title"的参数,并将其存储在一个名为"title"的字符串变量中。
7. String pubren = request.getParameter("pubren");
获取HTTP请求中名为"pubren"的参数,并将其存储在一个名为"pubren"的字符串变量中。
8. String laiyuan=request.getParameter("laiyuan");
获取HTTP请求中名为"laiyuan"的参数,并将其存储在一个名为"laiyuan"的字符串变量中。
9. String clickcount = request.getParameter("clickcount");
获取HTTP请求中名为"clickcount"的参数,并将其存储在一个名为"clickcount"的字符串变量中。
10. String dcontent = request.getParameter("dcontent");
获取HTTP请求中名为"dcontent"的参数,并将其存储在一个名为"dcontent"的字符串变量中。
11. String tupian2 = request.getParameter("tupian2");
获取HTTP请求中名为"tupian2"的参数,并将其存储在一个名为"tupian2"的字符串变量中。
12. String lanmuid = request.getParameter("lanmuid");
获取HTTP请求中名为"lanmuid"的参数,并将其存储在一个名为"lanmuid"的字符串变量中。
13. String lanmuming = request.getParameter("lanmuming");
获取HTTP请求中名为"lanmuming"的参数,并将其存储在一个名为"lanmuming"的字符串变量中。
14. String tuijian=request.getParameter("tuijian");
获取HTTP请求中名为"tuijian"的参数,并将其存储在一个名为"tuijian"的字符串变量中。
15. String hot=request.getParameter("hot");
获取HTTP请求中名为"hot"的参数,并将其存储在一个名为"hot"的字符串变量中。
16. String zuixin=request.getParameter("zuixin");
获取HTTP请求中名为"zuixin"的参数,并将其存储在一个名为"zuixin"的字符串变量中。
17. String zhaiyao=request.getParameter("zhaiyao");
获取HTTP请求中名为"zhaiyao"的参数,并将其存储在一个名为"zhaiyao"的字符串变量中。
18. String style=request.getParameter("style");
获取HTTP请求中名为"style"的参数,并将其存储在一个名为"style"的字符串变量中。
19. SimpleDateFormat sdfxinxi = new SimpleDateFormat("yyyy-MM-dd");
创建一个名为"sdfxinxi"的SimpleDateFormat对象,用于将日期格式化为"yyyy-MM-dd"的字符串。
20. xinxi.setZhaiyao(zhaiyao);
将"xinxi"对象的"zhaiyao"属性设置为"zhaiyao"变量的值。
21. xinxi.setTitle(title);
将"xinxi"对象的"title"属性设置为"title"变量的值。
22. xinxi.setPubren(pubren);
将"xinxi"对象的"pubren"属性设置为"pubren"变量的值。
23. xinxi.setLaiyuan(laiyuan);
将"xinxi"对象的"laiyuan"属性设置为"laiyuan"变量的值。
24. xinxi.setDcontent(dcontent);
将"xinxi"对象的"dcontent"属性设置为"dcontent"变量的值。
25. xinxi.setHot(hot!=null?1:0);
如果"hot"变量不为null,将"xinxi"对象的"hot"属性设置为1,否则设置为0。
26. xinxi.setTuijian(tuijian!=null?1:0);
如果"tuijian"变量不为null,将"xinxi"对象的"tuijian"属性设置为1,否则设置为0。
27. xinxi.setZuixin(zuixin!=null?1:0);
如果"zuixin"变量不为null,将"xinxi"对象的"zuixin"属性设置为1,否则设置为0。
28. xinxi.setTupian2(tupian2);
将"xinxi"对象的"tupian2"属性设置为"tupian2"变量的值。
29. xinxi.setLanmuid(new Integer(lanmuid));
将"xinxi"对象的"lanmuid"属性设置为"lanmuid"变量的值的整数形式。
30. xinxi.setLanmuming(lanmuming);
将"xinxi"对象的"lanmuming"属性设置为"lanmuming"变量的值。
31. DALBase.update(xinxi);
使用"xinxi"对象更新数据库中的记录。
32. try {
开始异常处理代码块。
33. if(style!=null&&style.equals("admin"))
如果"style"变量不为null,且其值为"admin",执行以下代码块。
34. response.sendRedirect("xinximanager.do?actiontype=get&seedid=201&lanmuid="+xinxi.getLanmuid());
将HTTP响应重定向到指定URL。
35. if(style!=null&&style.equals("huiyuan"))
如果"style"变量不为null,且其值为"huiyuan",执行以下代码块。
36. response.sendRedirect("../e/myxinximanager.jsp?seedid=m2");
将HTTP响应重定向到指定URL。
37. } catch (IOException e) {
捕获IOException异常。
38. e.printStackTrace();
输出异常堆栈跟踪信息。
39. }
异常处理代码块结束。
request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); int id = Integer.parseInt(request.getParameter("id")); String name = request.getParameter("name"); String description = request.getParameter("description"); String price = request.getParameter("price"); String category = request.getParameter("category"); String manufacturer = request.getParameter("manufacturer"); String stockQuantity = request.getParameter("stockQuantity"); String isAvailable = request.getParameter("isAvailable"); Product product = new Product(id, name, description, Double.parseDouble(price), category, manufacturer, Integer.parseInt(stockQuantity), null, LocalDateTime.now(), isAvailable); ProductDao productDao = new ProductDao(); int update = productDao.update(product); if(update > 0){ request.getRequestDispatcher("productListServlet").forward(request,response);添加注释
这段代码是一个Servlet中的doPost方法的代码片段,主要作用是根据请求中的参数更新商品信息,并将请求转发到商品列表页面。注释可以如下添加:
```
// 设置请求和响应的编码格式为UTF-8
request.setCharacterEncoding("utf-8");
response.setContentType("text/html;charset=utf-8");
// 从请求中获取商品的各个属性值
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
String description = request.getParameter("description");
String price = request.getParameter("price");
String category = request.getParameter("category");
String manufacturer = request.getParameter("manufacturer");
String stockQuantity = request.getParameter("stockQuantity");
String isAvailable = request.getParameter("isAvailable");
// 创建一个Product对象,用于更新商品信息
Product product = new Product(id, name, description, Double.parseDouble(price), category, manufacturer,
Integer.parseInt(stockQuantity), null, LocalDateTime.now(), isAvailable);
// 创建一个ProductDao对象,用于更新商品信息
ProductDao productDao = new ProductDao();
// 更新商品信息,并获取更新结果
int update = productDao.update(product);
// 如果更新成功,就将请求转发到商品列表页面
if(update > 0){
request.getRequestDispatcher("productListServlet").forward(request,response);
}
```
相关推荐
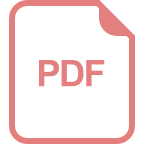
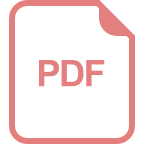













