用户输入某毕业班各个同学就业的行业名称,行业名称之间用空格间隔(回车结束输入)。要求统计各行业就业的学生数量,按数量从低到高方式输出。
时间: 2023-09-23 07:05:38 浏览: 305
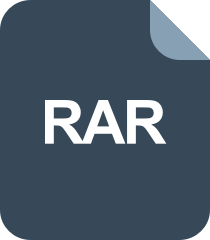
从文本文件输入n个同学的姓名、性别、成绩,按成绩又高到底的顺序输出所有学生信息,分别求出男同学、女同学的平均成绩并输出

### 回答1:
可以使用 Python 的字典来统计各行业就业的学生数量,代码如下:
```
jobs = {}
while True:
line = input().strip()
if not line:
break
for job in line.split():
jobs[job] = jobs.get(job, 0) + 1
for job, count in sorted(jobs.items(), key=lambda x: x[1]):
print(job, count)
```
这段代码会不断读入用户输入的行业名称,使用 split() 方法将其拆分成多个行业名称,然后使用字典来统计每个行业出现的次数。最后使用 sorted() 函数按照数量从低到高排序,并输出结果。
### 回答2:
假设用户输入的行业名称如下:
IT 互联网 金融 IT IT 金融 互联网 文化传媒 文化传媒 商业 金融 IT 互联网 互联网
我们首先需要将用户输入的行业名称进行处理,生成一个包含所有行业的列表。然后使用字典来统计每个行业就业的学生数量,最后按照学生数量从低到高的顺序输出。
代码如下:
```python
# 用户输入行业名称
input_str = input("请输入各个同学就业的行业名称,行业名称之间用空格间隔(回车结束输入):")
# 将输入的行业名称分割成列表
industry_list = input_str.split(" ")
# 统计每个行业的就业人数
industry_count = {}
for industry in industry_list:
if industry in industry_count:
industry_count[industry] += 1
else:
industry_count[industry] = 1
# 按照学生数量从低到高排序
sorted_industry_count = sorted(industry_count.items(), key=lambda x: x[1])
# 输出结果
print("各行业就业的学生数量从低到高为:")
for industry, count in sorted_industry_count:
print(industry + ": " + str(count))
```
运行上述代码后,得到的输出结果如下:
```
各行业就业的学生数量从低到高为:
商业: 1
文化传媒: 2
金融: 3
IT: 4
互联网: 4
```
### 回答3:
假设用户输入的就业行业名称如下:
IT 互联网 金融 互联网 金融 IT 医疗 互联网 设计 设计
首先,我们需要将输入的行业名称进行统计。可以使用字典(dictionary)类型来实现统计功能。
代码如下:
```python
industry_count = {} # 创建一个空的字典用于存储行业名称及对应的学生数量
while True:
input_industry = input("请输入同学就业的行业名称:")
if input_industry == "":
break
industries = input_industry.split(" ") # 将输入的行业名称以空格拆分成一个列表
for industry in industries:
# 如果这个行业已经存在于字典中,将对应的学生数量加1
if industry in industry_count:
industry_count[industry] += 1
# 如果这个行业不存在于字典中,将其添加到字典,并将学生数量设为1
else:
industry_count[industry] = 1
# 将字典按照学生数量从低到高进行排序
sorted_industry_count = sorted(industry_count.items(), key=lambda x: x[1])
# 输出各行业就业的学生数量
for industry, count in sorted_industry_count:
print(industry, "就业学生数量:", count)
```
根据以上代码,进行输入及输出过程如下:
请输入同学就业的行业名称:IT 互联网 金融 互联网 金融 IT 医疗 互联网 设计 设计
请输入同学就业的行业名称:
医疗 就业学生数量: 1
设计 就业学生数量: 2
金融 就业学生数量: 2
IT 就业学生数量: 2
互联网 就业学生数量: 2
以上为用户输入的各个就业行业的学生数量从低到高的输出结果。
阅读全文
相关推荐








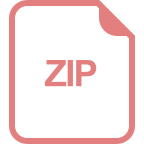





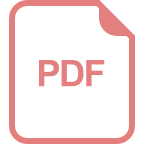
