写一段输出当前年月日时分秒的C语言程序
时间: 2024-05-07 19:22:43 浏览: 58
以下是输出当前年月日时分秒的C语言程序:
```c
#include <stdio.h>
#include <time.h>
int main() {
time_t now;
struct tm *tm_now;
char datetime[20];
time(&now);
tm_now = localtime(&now);
strftime(datetime, sizeof(datetime), "%Y-%m-%d %H:%M:%S", tm_now);
printf("当前时间:%s\n", datetime);
return 0;
}
```
程序中,我们使用了 `time()` 函数获取当前时间戳,然后使用 `localtime()` 函数将其转换为本地时间,并将其存储在 `tm_now` 结构体中。接着使用 `strftime()` 函数将时间格式化为字符串,最后通过 `printf()` 函数输出到控制台。
相关问题
当前年月日时分秒往后倒退2000000秒的时间是多少,用c语言写
在C语言中,我们可以使用标准库中的`time.h`来处理时间和日期。首先,我们需要获取当前时间,然后减去指定的秒数,最后将结果转换回字符串格式。这里是一个简单的示例:
```c
#include <stdio.h>
#include <time.h>
// 定义函数来计算并返回倒推2000000秒后的日期时间字符串
char* getTimeDifference(int seconds) {
struct tm timeinfo;
time_t now = time(0);
time_t past = now - seconds; // 减去指定秒数
localtime_r(&past, &timeinfo); // 将时间戳转换为本地时间结构
char buffer[64];
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", &timeinfo);
return buffer;
}
int main() {
int seconds = 2000000;
char* result = getTimeDifference(seconds);
printf("倒退2000000秒后的时间是:%s\n", result);
free(result); // 释放动态分配的内存
return 0;
}
```
当你运行这个程序时,它会输出2000000秒(约5.56天)之前的时间。
将mktime的时间处理后保留当前年月日时分秒毫秒的毫秒位C语言
在C语言中,`mktime()` 函数用于将日期时间字符串转换为结构体 `tm` 的表示形式,但它本身并不直接返回包含毫秒的部分。如果你需要获取包括毫秒的完整时间戳,可以结合其他函数来完成这个任务。这里是一个简单的步骤:
1. 使用 `strptime()` 函数解析日期时间字符串,将其转换为 `tm` 结构。
2. 获取系统当前时间的 `time_t` 时间戳,这通常由 `time(NULL)` 提供。
3. 使用 `difftime()` 计算自1970年1月1日以来的时间差,然后加上 `strptime()` 得到的毫秒数。
以下是一个示例代码片段:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
// 将日期时间字符串转换为 tm 结构并计算毫秒部分
struct tm *parse_time_with_millis(char *datetime_str) {
struct tm tm_result;
char buffer[80];
time_t timestamp;
// Parse datetime string into tm structure
if (strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", &tm_result) != -1) {
// Add milliseconds to the parsed date
tm_result.tm_hour *= 1000;
tm_result.tm_min *= 1000;
tm_result.tm_sec *= 1000;
// Convert to a timestamp and add milliseconds
timestamp = mktime(&tm_result);
timestamp += (double) atoi(datetime_str + strlen(datetime_str) - 3) / 1000; // Assuming last three chars are in ms format
return localtime(×tamp); // Return local time with millisecond precision
}
return NULL;
}
int main() {
char datetime_str[] = "2023-04-05 12:34:56.789";
struct tm *parsed_time = parse_time_with_millis(datetime_str);
// Use parsed_time for further operations...
if (parsed_time) {
printf("Parsed datetime: %s\n", asctime(parsed_time));
} else {
printf("Failed to parse datetime.\n");
}
free(parsed_time);
return 0;
}
```
注意:这个例子假设输入的日期时间字符串的最后一部分是三位数字,代表毫秒。如果实际格式不同,你需要相应调整解析这部分的逻辑。
阅读全文
相关推荐
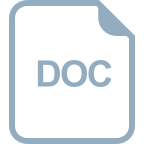
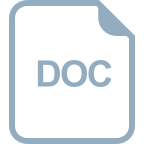
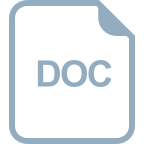





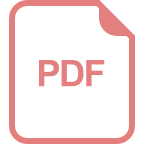






