参照《重构-改善既有代码的设计》书中关于不良设计(违反SOLID)、不良代码(代码坏味道bad smells)的判别标准进行重构void Library::filemanage() { fstream f; string s; f.open("books.txt", ios::out); addBook(); for (int i = 0; i < books.size(); i++) { f << books[i]->getName() << " " << books[i]->getAuther() << " " << books[i]->getNum() << " " << books[i]->getinstock() << endl; } f.close(); cout << "输入成功" << endl; } void Library::open() { fstream f; f.open("books.txt", ios::in); string name; string auther; string number; int instock; while (f >> name >> auther >> number >> instock) { cout << name << " " << auther << " " << number << " " << instock << endl; int i; for (i = 0; i < books.size(); i++) { if (books[i]->getNum() == number) { books[i]->setname(name); books[i]->setauther(auther); books[i]->setnum(number); books[i]->set(instock); } } } f.close(); cout << "输出成功" << endl; system("pause"); system("cls"); }
时间: 2024-03-17 14:41:05 浏览: 137
以下一个可能的重构方案:
1. 单一职责原则:将文件读写和书籍管理分离成两个类,`FileManager` 和 `BookManager`。
```c++
class FileManager {
public:
virtual ~FileManager() {}
virtual void write(const std::string& filename, const std::vector<std::string>& lines) = 0;
virtual std::vector<std::string> read(const std::string& filename) = 0;
};
class BookManager {
public:
virtual ~BookManager() {}
virtual bool addBook(const std::string& name, const std::string& author, const std::string& num, int instock) = 0;
virtual std::vector<Book*> getBooks() = 0;
};
```
2. 依赖倒置原则:将 `Library` 类中的文件读写操作抽象成 `FileManager` 接口,并将其作为 `Library` 的构造函数参数传入。
```c++
class Library {
public:
Library(FileManager* fileManager, BookManager* bookManager);
bool addBook(const std::string& name, const std::string& author, const std::string& num, int instock);
void printBooks();
private:
FileManager* fileManager_;
BookManager* bookManager_;
};
```
3. 开闭原则:使用工厂模式来创建 `Book` 对象,以便将来添加或删除书籍信息的属性时不需要修改 `Book` 类。
```c++
class BookFactory {
public:
static Book* create(const std::string& name, const std::string& author, const std::string& num, int instock);
};
```
4. 代码坏味道:将文件读写操作抽象成独立的函数,并将其封装在 `FileManager` 类中。
```c++
class TextFileManager : public FileManager {
public:
void write(const std::string& filename, const std::vector<std::string>& lines) override;
std::vector<std::string> read(const std::string& filename) override;
};
void TextFileManager::write(const std::string& filename, const std::vector<std::string>& lines) {
std::ofstream f(filename);
for (const auto& line : lines) {
f << line << std::endl;
}
}
std::vector<std::string> TextFileManager::read(const std::string& filename) {
std::ifstream f(filename);
std::vector<std::string> lines;
std::string line;
while (std::getline(f, line)) {
lines.push_back(line);
}
return lines;
}
```
重构后的 `Library` 类中的 `addBook()` 和 `printBooks()` 函数的实现如下:
```c++
bool Library::addBook(const std::string& name, const std::string& author, const std::string& num, int instock) {
bool success = bookManager_->addBook(name, author, num, instock);
if (success) {
std::vector<std::string> lines;
for (const auto& book : bookManager_->getBooks()) {
lines.push_back(book->getName() + " " + book->getAuthor() + " " + book->getNum() + " " + std::to_string(book->getInstock()));
}
fileManager_->write("books.txt", lines);
}
return success;
}
void Library::printBooks() {
std::vector<std::string> lines = fileManager_->read("books.txt");
for (const auto& line : lines) {
std::istringstream iss(line);
std::string name, author, num;
int instock;
iss >> name >> author >> num >> instock;
std::cout << name << " " << author << " " << num << " " << instock << std::endl;
}
}
```
阅读全文
相关推荐
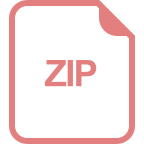
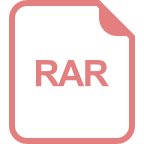
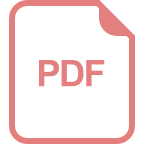
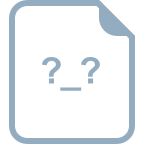

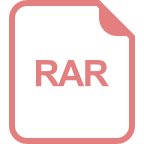
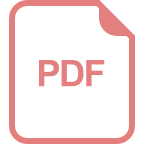
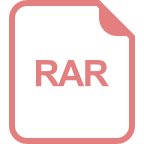
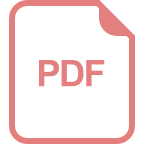
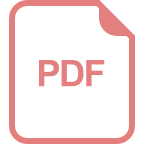
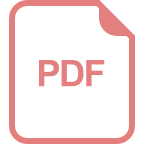
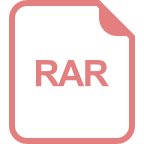
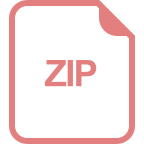
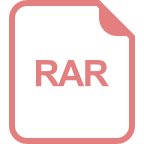
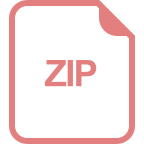
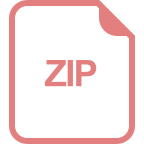