vs使用freeglut编程实现四连通边界填算法及泛滥填充算法
时间: 2023-07-26 12:10:30 浏览: 76
首先,你需要在 Visual Studio 中安装 FreeGLUT 库。可以在官网下载对应版本的库,并按照官方指南进行安装。
接下来,你可以创建一个空的 C++ 项目,并在项目属性中添加 FreeGLUT 库的链接。具体步骤如下:
1. 右键点击项目,选择“属性”。
2. 在左侧面板中选择“VC++ 目录”。
3. 在“包含目录”中添加 FreeGLUT 的 include 文件夹路径。
4. 在“库目录”中添加 FreeGLUT 的 lib 文件夹路径。
5. 在“链接器 -> 输入 -> 附加依赖项”中添加 FreeGLUT 的 lib 文件名(例如:freeglut.lib)。
完成上述步骤后,你可以开始编写四连通边界填算法和泛滥填充算法的代码。以下是一个简单的示例:
```cpp
#include <GL/freeglut.h>
void drawPixel(int x, int y) {
glBegin(GL_POINTS);
glVertex2i(x, y);
glEnd();
}
void boundaryFill4(int x, int y, float* fillColor, float* borderColor) {
float interiorColor[3];
glReadPixels(x, y, 1, 1, GL_RGB, GL_FLOAT, interiorColor);
if (interiorColor[0] != borderColor[0] ||
interiorColor[1] != borderColor[1] ||
interiorColor[2] != borderColor[2]) {
drawPixel(x, y);
glFlush();
boundaryFill4(x + 1, y, fillColor, borderColor);
boundaryFill4(x - 1, y, fillColor, borderColor);
boundaryFill4(x, y + 1, fillColor, borderColor);
boundaryFill4(x, y - 1, fillColor, borderColor);
}
}
void floodFill(int x, int y, float* fillColor, float* borderColor) {
float interiorColor[3];
glReadPixels(x, y, 1, 1, GL_RGB, GL_FLOAT, interiorColor);
if (interiorColor[0] != borderColor[0] ||
interiorColor[1] != borderColor[1] ||
interiorColor[2] != borderColor[2]) {
drawPixel(x, y);
glFlush();
floodFill(x + 1, y, fillColor, borderColor);
floodFill(x - 1, y, fillColor, borderColor);
floodFill(x, y + 1, fillColor, borderColor);
floodFill(x, y - 1, fillColor, borderColor);
}
}
void display() {
float fillColor[] = { 1.0, 1.0, 0.0 };
float borderColor[] = { 0.0, 0.0, 0.0 };
glClearColor(1.0, 1.0, 1.0, 1.0);
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(0.0, 0.0, 0.0);
glBegin(GL_LINE_LOOP);
glVertex2i(100, 100);
glVertex2i(200, 100);
glVertex2i(200, 200);
glVertex2i(100, 200);
glEnd();
boundaryFill4(150, 150, fillColor, borderColor);
//floodFill(150, 150, fillColor, borderColor);
glFlush();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(400, 400);
glutCreateWindow("Boundary Fill and Flood Fill Algorithms");
gluOrtho2D(0, 400, 0, 400);
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
该示例中包含了两个函数:`boundaryFill4` 和 `floodFill`,它们分别实现了四连通边界填算法和泛滥填充算法。在 `display` 函数中,我们先画了一个矩形,并在其中心点处调用了这两个函数。
注意,该示例中只实现了单色填充。如果你需要用不同的颜色填充不同区域,可以在 `drawPixel` 函数中添加更多参数,并在 `boundaryFill4` 和 `floodFill` 函数中进行判断和处理。
相关推荐
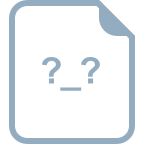
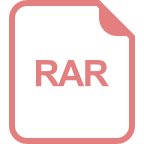














