职工工资管理系统的设计与实现(顺序表实现)
时间: 2023-08-14 17:03:48 浏览: 54
职工工资管理系统是一个涉及到多个功能的复杂系统,可以通过顺序表实现。下面是顺序表实现职工工资管理系统的步骤:
1. 定义职工结构体
首先,我们需要定义一个职工结构体,该结构体包含职工的基本信息,如职工编号、姓名、性别、职位、工资等字段。
```c
typedef struct Employee {
int id; // 职工编号
char name[20]; // 姓名
char gender[5]; // 性别
char position[20]; // 职位
float salary; // 工资
} Employee;
```
2. 定义顺序表结构体
接下来,我们需要定义一个顺序表结构体,用于存储职工信息。顺序表结构体包含一个指向职工结构体数组的指针,以及当前职工数量和最大职工数量两个字段。
```c
#define MAX_EMPLOYEE_NUM 100 // 最大职工数量
typedef struct EmployeeList {
Employee* employees; // 职工数组指针
int count; // 当前职工数量
int max; // 最大职工数量
} EmployeeList;
```
3. 初始化顺序表
在定义好顺序表结构体后,我们需要编写一个函数来初始化顺序表。该函数需要动态分配内存空间,并将当前职工数量初始化为0,最大职工数量初始化为预设值。同时,需要检查内存是否分配成功,分配成功后将职工数组指针指向分配的内存空间。
```c
void InitEmployeeList(EmployeeList* list) {
list->employees = (Employee*)malloc(sizeof(Employee) * MAX_EMPLOYEE_NUM);
if (list->employees == NULL) {
printf("Error: out of memory!\n");
exit(1);
}
list->count = 0;
list->max = MAX_EMPLOYEE_NUM;
}
```
4. 添加职工信息
添加职工信息是职工工资管理系统中最基本的功能。我们需要编写一个函数来实现添加职工信息的功能。该函数需要检查当前职工数量是否已达到最大值,如果已经达到最大值则无法添加新的职工信息。如果当前职工数量没有达到最大值,则需要依次输入新职工的编号、姓名、性别、职位和工资等信息,并将这些信息存储到职工数组中。
```c
void AddEmployee(EmployeeList* list) {
if (list->count >= list->max) {
printf("Error: the employee list is full!\n");
return;
}
Employee newEmployee;
printf("Please enter the employee ID: ");
scanf("%d", &newEmployee.id);
printf("Please enter the employee name: ");
scanf("%s", newEmployee.name);
printf("Please enter the employee gender: ");
scanf("%s", newEmployee.gender);
printf("Please enter the employee position: ");
scanf("%s", newEmployee.position);
printf("Please enter the employee salary: ");
scanf("%f", &newEmployee.salary);
list->employees[list->count++] = newEmployee;
printf("Add employee success!\n");
}
```
5. 查找职工信息
查找职工信息是职工工资管理系统中常用的功能之一。我们需要编写一个函数来实现根据职工编号查找职工信息的功能。该函数需要依次输入职工编号,并遍历职工数组,查找与输入的职工编号相同的职工信息。如果找到了相应的职工信息,则输出该职工的所有信息。否则,提示用户没有找到该职工信息。
```c
void FindEmployee(EmployeeList* list) {
int id;
printf("Please enter the employee ID: ");
scanf("%d", &id);
for (int i = 0; i < list->count; i++) {
if (list->employees[i].id == id) {
printf("ID: %d\n", list->employees[i].id);
printf("Name: %s\n", list->employees[i].name);
printf("Gender: %s\n", list->employees[i].gender);
printf("Position: %s\n", list->employees[i].position);
printf("Salary: %.2f\n", list->employees[i].salary);
return;
}
}
printf("Error: no such employee!\n");
}
```
6. 修改职工信息
修改职工信息是职工工资管理系统中常用的功能之一。我们需要编写一个函数来实现根据职工编号修改职工信息的功能。该函数需要依次输入职工编号,并遍历职工数组,查找与输入的职工编号相同的职工信息。如果找到了相应的职工信息,则依次输入需要修改的职工信息,并将修改后的信息存储到职工数组中。否则,提示用户没有找到该职工信息。
```c
void ModifyEmployee(EmployeeList* list) {
int id;
printf("Please enter the employee ID: ");
scanf("%d", &id);
for (int i = 0; i < list->count; i++) {
if (list->employees[i].id == id) {
printf("Please enter the employee name: ");
scanf("%s", list->employees[i].name);
printf("Please enter the employee gender: ");
scanf("%s", list->employees[i].gender);
printf("Please enter the employee position: ");
scanf("%s", list->employees[i].position);
printf("Please enter the employee salary: ");
scanf("%f", &list->employees[i].salary);
printf("Modify employee success!\n");
return;
}
}
printf("Error: no such employee!\n");
}
```
7. 删除职工信息
删除职工信息是职工工资管理系统中常用的功能之一。我们需要编写一个函数来实现根据职工编号删除职工信息的功能。该函数需要依次输入职工编号,并遍历职工数组,查找与输入的职工编号相同的职工信息。如果找到了相应的职工信息,则将该职工信息从职工数组中删除。否则,提示用户没有找到该职工信息。
```c
void DeleteEmployee(EmployeeList* list) {
int id;
printf("Please enter the employee ID: ");
scanf("%d", &id);
for (int i = 0; i < list->count; i++) {
if (list->employees[i].id == id) {
for (int j = i + 1; j < list->count; j++) {
list->employees[j - 1] = list->employees[j];
}
list->count--;
printf("Delete employee success!\n");
return;
}
}
printf("Error: no such employee!\n");
}
```
8. 显示职工信息
显示职工信息是职工工资管理系统中常用的功能之一。我们需要编写一个函数来实现显示所有职工信息的功能。该函数需要遍历职工数组,依次输出每个职工的所有信息。
```c
void DisplayEmployeeList(EmployeeList* list) {
printf("ID\t Name\t Gender\t Position\t Salary\n");
for (int i = 0; i < list->count; i++) {
printf("%d\t %s\t %s\t %s\t %.2f\n", list->employees[i].id, list->employees[i].name,
list->employees[i].gender, list->employees[i].position, list->employees[i].salary);
}
}
```
9. 释放内存空间
在程序结束时,需要释放动态分配的内存空间。我们需要编写一个函数来实现释放内存空间的功能。
```c
void DestroyEmployeeList(EmployeeList* list) {
free(list->employees);
list->count = 0;
list->max = 0;
}
```
以上就是使用顺序表实现职工工资管理系统的步骤。需要注意的是,实际应用中可能还需要实现其他功能,如数据的存储和读取、数据的排序和查找等。
相关推荐
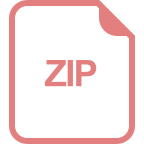
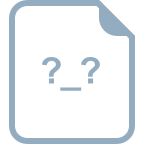
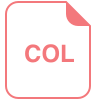
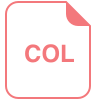
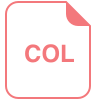
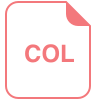
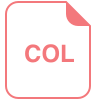









