用顺序表实现电影院售票管理系统的设计与实现
时间: 2023-08-14 17:03:48 浏览: 97
好的,我明白了。顺序表是一种常见的数据结构,可以用来实现电影院售票管理系统的设计与实现。
首先,我们需要定义一个电影信息的数据结构,其中包括电影的名称、导演、主演、上映时间等信息。可以使用一个结构体来表示:
```
struct Movie {
char name[50];
char director[50];
char actor[50];
char releaseDate[20];
};
```
接下来,我们需要定义一个顺序表的数据结构,用来存储电影的信息。顺序表的核心是一个动态数组,它包括存储电影信息的结构体和数组的长度。
```
struct SeqList {
struct Movie *movies;
int length;
};
```
有了这个顺序表结构体,我们就可以开始实现电影院售票管理系统了。我们可以定义一些函数来实现对顺序表的基本操作,例如:
1. 初始化顺序表
```
void initSeqList(struct SeqList *seqList) {
seqList->movies = NULL;
seqList->length = 0;
}
```
2. 插入元素
```
void insertElement(struct SeqList *seqList, struct Movie movie) {
seqList->length++;
seqList->movies = (struct Movie*)realloc(seqList->movies, seqList->length * sizeof(struct Movie));
seqList->movies[seqList->length-1] = movie;
}
```
3. 删除元素
```
void deleteElement(struct SeqList *seqList, char *name) {
int i;
for (i = 0; i < seqList->length; i++) {
if (strcmp(seqList->movies[i].name, name) == 0) {
int j;
for (j = i; j < seqList->length-1; j++) {
seqList->movies[j] = seqList->movies[j+1];
}
seqList->length--;
seqList->movies = (struct Movie*)realloc(seqList->movies, seqList->length * sizeof(struct Movie));
return;
}
}
}
```
4. 查找元素
```
struct Movie* findElement(struct SeqList *seqList, char *name) {
int i;
for (i = 0; i < seqList->length; i++) {
if (strcmp(seqList->movies[i].name, name) == 0) {
return &seqList->movies[i];
}
}
return NULL;
}
```
5. 修改元素
```
void updateElement(struct Movie *movie, char *director, char *actor, char *releaseDate) {
strcpy(movie->director, director);
strcpy(movie->actor, actor);
strcpy(movie->releaseDate, releaseDate);
}
```
有了这些基本操作,我们就可以实现电影院售票管理系统了。例如,我们可以定义一个函数来添加电影:
```
void addMovie(struct SeqList *seqList) {
struct Movie movie;
printf("Enter movie name: ");
scanf("%s", movie.name);
printf("Enter director name: ");
scanf("%s", movie.director);
printf("Enter actor name: ");
scanf("%s", movie.actor);
printf("Enter release date: ");
scanf("%s", movie.releaseDate);
insertElement(seqList, movie);
printf("Movie added successfully.\n");
}
```
类似地,我们可以定义其他函数来实现对电影院售票管理系统的其他操作。
阅读全文
相关推荐
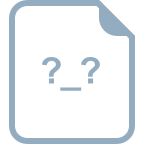



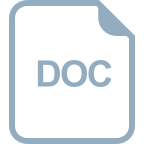

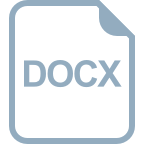
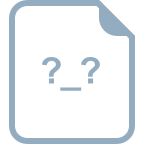
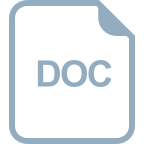
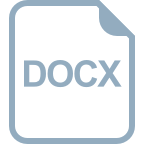
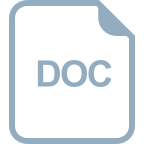
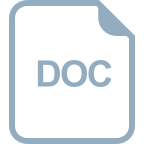
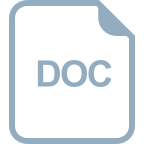
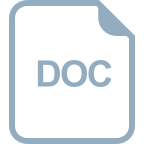
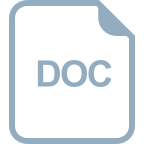
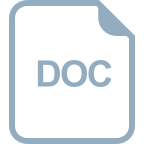