编写一个程序,使堆栈储存100个学生记录用java
时间: 2023-08-24 12:07:22 浏览: 93
好的,下面是使用 Java 语言实现的一个可以储存100个学生记录的堆栈的示例程序。
```java
import java.util.EmptyStackException;
class Student {
String name;
int age;
String major;
public Student(String name, int age, String major) {
this.name = name;
this.age = age;
this.major = major;
}
@Override
public String toString() {
return name + ", " + age + ", " + major;
}
}
class Stack {
private final int MAX_SIZE = 100;
private final Student[] stackArray = new Student[MAX_SIZE];
private int top = -1;
public boolean isEmpty() {
return top == -1;
}
public boolean isFull() {
return top == MAX_SIZE - 1;
}
public void push(Student student) {
if (isFull()) {
System.out.println("Stack overflow!");
return;
}
top++;
stackArray[top] = student;
}
public void pop() {
if (isEmpty()) {
throw new EmptyStackException();
}
top--;
}
public Student peek() {
if (isEmpty()) {
throw new EmptyStackException();
}
return stackArray[top];
}
}
public class Main {
public static void main(String[] args) {
Stack studentStack = new Stack();
// push 100 student records into the stack
for (int i = 1; i <= 100; i++) {
Student student = new Student("Student " + i, 18 + i % 5, "Major " + i % 10);
studentStack.push(student);
}
// pop and print all the student records from the stack
while (!studentStack.isEmpty()) {
Student student = studentStack.peek();
System.out.println(student);
studentStack.pop();
}
}
}
```
这个程序使用了一个类`Student`来储存每个学生的记录,其中包括姓名、年龄和专业。堆栈的容量被设为100,使用一个类`Stack`来实现堆栈的基本操作,包括`push`、`pop`、`peek`等。在`main`函数中,我们向堆栈中压入100个学生记录,然后依次弹出并打印每个学生的记录。
希望这个示例程序能够帮到您,如有疑问请随时向我提问。
阅读全文
相关推荐
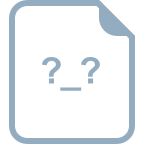
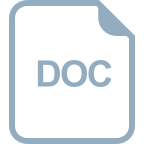
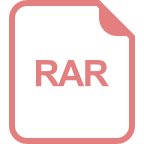

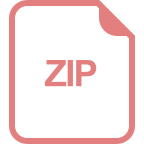
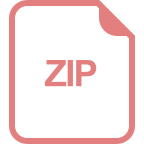
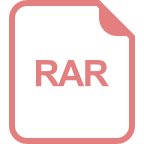
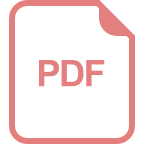
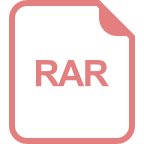
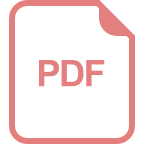
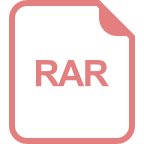
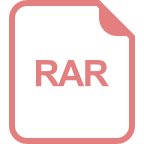
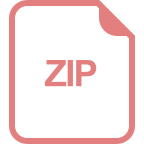
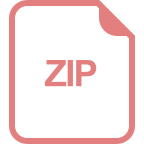
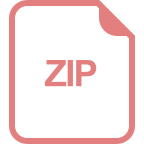
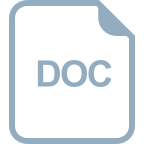
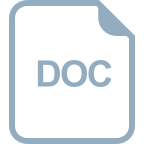
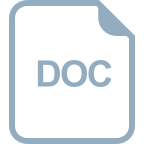