java从键盘输入一串数组,再输入一个数值,如果这个数值在数组中,输出数值在数组中的位置,否则返回-1
时间: 2024-09-22 13:08:53 浏览: 47
在Java中,你可以通过Scanner类获取用户的键盘输入,然后遍历数组来查找给定的值。这里是一个简单的示例程序:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 获取用户输入的一串整数,存储在一个数组里
System.out.println("请输入数组元素,用空格隔开:");
String input = scanner.nextLine();
int[] array = parseIntegers(input.split(" "));
// 输入一个数值
System.out.println("请输入一个数值:");
int searchValue = scanner.nextInt();
// 查找数值并输出位置
int position = findPosition(array, searchValue);
if (position != -1) {
System.out.printf("数值 %d 在数组中的位置是 %d\n", searchValue, position);
} else {
System.out.println("数值不在数组中,返回 -1");
}
scanner.close();
}
// 将字符串转换成整数数组
private static int[] parseIntegers(String[] strArray) {
int[] integers = new int[strArray.length];
for (int i = 0; i < strArray.length; i++) {
integers[i] = Integer.parseInt(strArray[i]);
}
return integers;
}
// 查找数组中数值的位置
private static int findPosition(int[] array, int value) {
for (int i = 0; i < array.length; i++) {
if (array[i] == value) {
return i; // 如果找到,返回索引
}
}
return -1; // 如果没找到,返回 -1
}
}
```
在这个程序中,首先读取用户的输入作为字符串数组,然后将字符串转换为整数数组。接着让用户输入一个数值,通过`findPosition`函数查找该值在数组中的位置。如果找到就返回位置,否则返回-1。
阅读全文
相关推荐






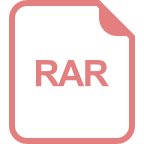
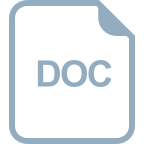










