boost.python多维列表传递给c++中的结构体
时间: 2023-10-16 08:30:17 浏览: 108
要将多维列表传递给C++中的结构体,可以使用Boost.Python库提供的`boost::python::list`和`boost::python::extract`类来访问Python中的列表,并使用C++中的结构体来保存列表的数据。
以下是一个示例代码,其中包括一个C++中的结构体`Person`和一个接受`Person`结构体的函数`process_person`,以及一个将多维列表转换为`Person`结构体并传递给`process_person`函数的Python脚本。
C++代码:
```cpp
#include <iostream>
#include <string>
#include <boost/python.hpp>
using namespace boost::python;
// 定义结构体
struct Person {
std::string name;
int age;
std::string gender;
};
// 定义函数,接受Person结构体
void process_person(const Person& person) {
std::cout << "Name: " << person.name << ", Age: " << person.age << ", Gender: " << person.gender << std::endl;
}
// 将多维列表转换为Person结构体并传递给process_person函数
void process_person_list(const list& person_list) {
int num_persons = len(person_list);
for (int i = 0; i < num_persons; ++i) {
// 从列表中提取元素
list person_info = extract<list>(person_list[i]);
std::string name = extract<std::string>(person_info[0]);
int age = extract<int>(person_info[1]);
std::string gender = extract<std::string>(person_info[2]);
// 创建Person结构体并调用process_person函数
Person person = {name, age, gender};
process_person(person);
}
}
// 导出模块
BOOST_PYTHON_MODULE(example) {
def("process_person_list", process_person_list);
}
```
Python代码:
```python
import example
# 定义多维列表
person_list = [
['Alice', 25, 'Female'],
['Bob', 30, 'Male'],
['Charlie', 35, 'Male']
]
# 将多维列表传递给C++中的process_person_list函数
example.process_person_list(person_list)
```
运行Python脚本后,输出结果:
```
Name: Alice, Age: 25, Gender: Female
Name: Bob, Age: 30, Gender: Male
Name: Charlie, Age: 35, Gender: Male
```
阅读全文
相关推荐
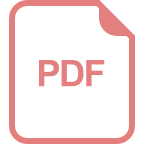
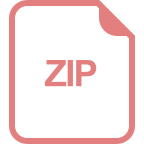
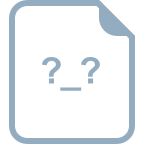


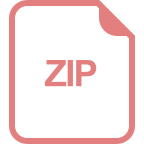
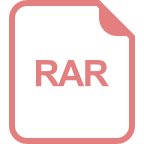
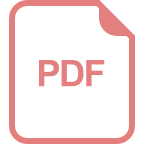
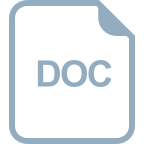
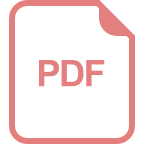
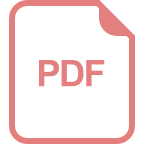
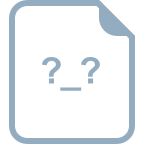
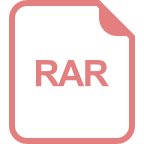
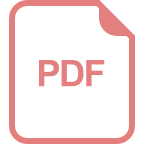
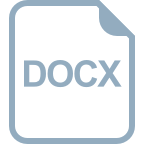
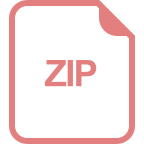