paillier算法实现java代码
时间: 2024-12-14 09:07:03 浏览: 10
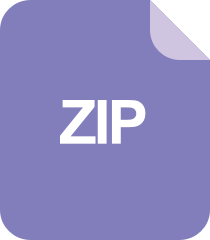
Paillier算法原理与实现_密码学源代码_C语言程序_C++程序源代码

Paillier公钥密码系统是一种非对称加密算法,主要用于保护数据隐私并支持加法和乘法操作。在Java中实现Paillier算法的基本步骤包括生成密钥对、加密、解密和同态运算。以下是简单的Java代码示例:
```java
import org.bouncycastle.crypto.params.ECPublicKeyParameters;
import org.bouncycastle.crypto.params.RSAPrivateCrtKeyParameters;
public class Paillier {
// 密钥生成
public static void generateKeys() {
ECPublicKeyParameters publicKey = ...; // 使用合适的库生成公钥
RSAPrivateCrtKeyParameters privateKey = ...; // 同样地,生成私钥
// 保存密钥对
...
}
// 加密函数
public static BigInteger encrypt(BigInteger plaintext, ECPublicKeyParameters publicKey) {
BigInteger n = publicKey.getModulus();
BigInteger g = publicKey.getQ().getWeylExponent();
BigInteger c1 = plaintext.modPow(g, n);
BigInteger r = new BigInteger(n.bitLength(), Math.random()).mod(n).add(1); // 随机数防止模n溢出
return c1.multiply(r).modPow(n, n); // 注意将结果取模n²
}
// 解密函数
public static BigInteger decrypt(BigInteger ciphertext, RSAPrivateCrtKeyParameters privateKey) {
BigInteger n = privateKey.getModulus();
BigInteger lambda = privateKey.getPrimeP().subtract(BigInteger.ONE).multiply(privateKey.getPrimeQ()).divide(privateKey.getPublicExponent());
BigInteger c1 = ciphertext.modPow(lambda, n).divide(ciphertext.mod(n));
BigInteger c0 = ciphertext.divide(r);
return c1.subtract(c0).mod(n);
}
// 同态加法
public static BigInteger homomorphicAdd(BigInteger a, BigInteger b, ECPublicKeyParameters publicKey) {
BigInteger encryptedA = encrypt(a, publicKey);
BigInteger encryptedB = encrypt(b, publicKey);
BigInteger sumEncrypted = encryptedA.add(encryptedB);
return decrypt(sumEncrypted, ...); // 解密返回加法结果
}
// 示例
public static void main(String[] args) {
generateKeys();
BigInteger plaintext = ...; // 待加密的明文
BigInteger encrypted = encrypt(plaintext, ...); // 使用公钥加密
BigInteger decrypted = decrypt(encrypted, ...); // 使用私钥解密
}
}
```
注意:这个代码片段使用了`bouncycastle`库,需要先引入该库。实际应用中,你需要处理更复杂的错误检查和异常情况。
阅读全文
相关推荐
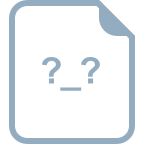
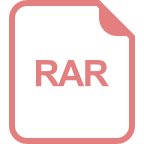
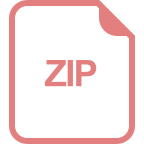
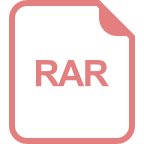
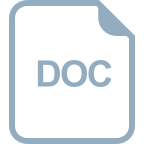
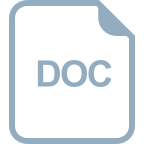
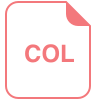
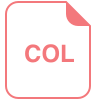
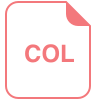
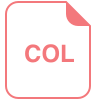
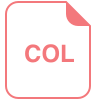
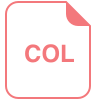


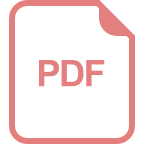
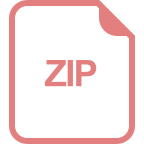
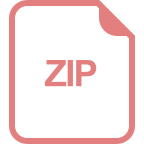