用C语言读入一行或多行由“ #”结尾的MINIC源程序,完成该段源程序的词法分析。 实验结果:输入任意的一段源程序,能分别按标识符、整常数、实常数、关键字和特殊符分类输出打印各单词的token形式。
时间: 2024-09-24 13:14:54 浏览: 28
在C语言中,对MINIC源程序进行词法分析通常会涉及到使用预处理器指令如`#include`、`#define`等。对于包含`#`的输入,你可以创建一个简单的程序,利用循环逐个字符处理,并结合一些状态机的概念来识别不同的token。
以下是一个简化的伪代码示例:
```c
#include <stdio.h>
#include <ctype.h>
typedef enum {
TOKEN_IDENTIFIER,
TOKEN_INTEGER,
TOKEN_REAL,
TOKEN_KEYWORD,
TOKEN_SPECIAL
} TokenType;
void analyze_source(char *input) {
char *current = input;
while (*current != '\0') {
if (isalpha(*current)) { // Identifier or keyword start
while (isalnum(*current)) {
current++;
}
// Check if it's a keyword or identifier
if (strcmp(current, "#") == 0) { // Special case for '#'
printf("TOKEN_SPECIAL: %s\n", input);
break;
} else {
// Assuming identifiers and keywords are stored in some predefined list
if (check_keyword(current)) {
printf("TOKEN_KEYWORD: %s\n", current);
} else {
printf("TOKEN_IDENTIFIER: %s\n", current);
}
}
current++; // Skip over the last character
} else if (isdigit(*current)) { // Integer or real number
while (isdigit(*current)) {
current++;
}
// Similar logic to handle integers and reals
// Check if the next character is '.'
if (*current == '.') {
current++; // Skip '.'
while (isdigit(*current)) {
current++;
}
}
// Handle integer or real constant output
} else if (*current == '#') { // End of line comment
while (*current != '\n') {
current++;
}
current++; // Skip newline
} else { // Other special characters like ', ;'
printf("TOKEN_SPECIAL: %c\n", *current);
current++;
}
}
}
int main() {
char source[100];
fgets(source, sizeof(source), stdin);
analyze_source(source);
return 0;
}
```
相关推荐
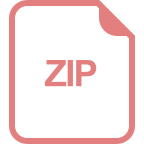
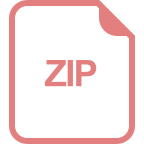
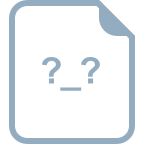














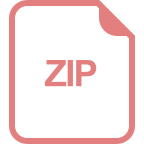