java读取excel复杂表头
时间: 2023-09-03 20:11:17 浏览: 143
Java读取Excel的表头可以使用Apache POI库来实现。对于复杂表头,可以使用递归的方式进行处理。下面是一个示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.InputStream;
public class ExcelReader {
public static void main(String[] args) {
try {
InputStream inp = new FileInputStream("path/to/your/excel/file.xlsx");
Workbook workbook = new XSSFWorkbook(inp);
Sheet sheet = workbook.getSheetAt(0); // 读取第一个sheet页
int rowIndex = 0; // 表头所在的行索引
Row headerRow = sheet.getRow(rowIndex);
// 读取表头
readHeader(headerRow, 0, "");
workbook.close();
inp.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private static void readHeader(Row row, int colIndex, String columnHeader) {
Cell cell = row.getCell(colIndex);
String value = cell.getStringCellValue();
if (cell.getCellType() == CellType.BLANK) {
return;
}
// 处理合并单元格
int mergedRegionIndex = findMergedRegionIndex(row.getSheet(), row.getRowNum(), colIndex);
if (mergedRegionIndex >= 0) {
value = row.getSheet().getMergedRegion(mergedRegionIndex).getCell(0).getStringCellValue();
}
columnHeader += value + " ";
// 递归处理子列
if (cell.getCellType() == CellType.STRING && cell.getStringCellValue().contains(" ")) {
readHeader(row, colIndex + 1, columnHeader);
} else {
System.out.println(columnHeader.trim());
}
}
private static int findMergedRegionIndex(Sheet sheet, int rowIndex, int colIndex) {
for (int i = 0; i < sheet.getNumMergedRegions(); i++) {
CellRangeAddress range = sheet.getMergedRegion(i);
if (range.isInRange(rowIndex, colIndex)) {
return i;
}
}
return -1;
}
}
```
这段代码会读取Excel文件中第一个sheet页的第一行作为表头,并且会处理合并单元格的情况。对于复杂表头,会使用递归的方式处理子列。每读取到一个完整的表头,会打印出来。你可以根据需要对表头进行其他的处理操作。请将代码中的"path/to/your/excel/file.xlsx"替换为你实际的Excel文件路径。
阅读全文
相关推荐








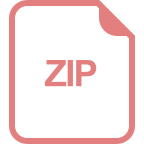






