pipeline java
时间: 2024-08-13 14:03:13 浏览: 26
Pipeline in Java refers to the concept of creating a sequence of operations that can be executed one after another to process data. It's commonly used in functional programming and Apache Beam, a distributed processing framework, where it enables developers to define complex data processing pipelines as a series of stages.
Here's a simple example of how you might use a pipeline in Java:
```java
import org.apache.beam.sdk.Pipeline;
import org.apache.beam.sdk.io.TextIO;
import org.apache.beam.sdk.options.PipelineOptionsFactory;
import org.apache.beam.sdk.transforms.DoFn;
import org.apache.beam.sdk.transforms.ParDo;
public class WordCount {
public static void main(String[] args) {
// Create a new Pipeline options instance
PipelineOptions options = PipelineOptionsFactory.create();
// Create a new Pipeline with the given options
Pipeline pipeline = Pipeline.create(options);
// Read input text from a file
String input = "input.txt";
PCollection<String> lines = pipeline.read(TextIO.from(input));
// Apply a ParDo transform to tokenize the words
PCollection<String> words = lines.apply(ParDo.of(new TokenizerFn()));
// Count the occurrences of each word
PCollection<KV<String, Long>> counts = words.apply(Count.perElement());
// Write the result to an output file
String output = "output.txt";
counts.writeTo(TextIO.to(output));
// Run the pipeline
pipeline.run().waitUntilFinish();
}
}
// Custom DoFn for tokenizing words
class TokenizerFn extends DoFn<String, String> {
@ProcessElement
public void process(@Element String line, OutputReceiver<String> receiver) {
String[] tokens = line.split("\\s+");
for (String token : tokens) {
receiver.output(token);
}
}
}
```
In this example, a `Pipeline` is created, which reads text from a file, tokenizes the words using a custom `TokenizerFn`, counts the occurrences of each word, and finally writes the results to an output file.
相关推荐
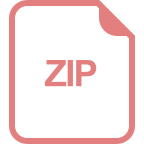
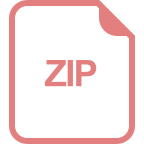
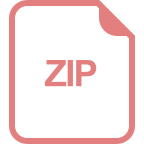








