const char* 转python str
时间: 2023-11-13 19:03:45 浏览: 187
您好,请问您是要将 C++ 中的 const char* 类型转换为 Python 中的 str 类型吗?若是的话,您可以使用 Python 中的 ctypes 模块。具体实现代码如下:
```python
import ctypes
# 定义 const char* 类型的变量
c_str = b"hello world"
# 将 c_str 转换为 Python 中的 str 类型
py_str = ctypes.c_char_p(c_str).value.decode('utf-8')
print(py_str) # 输出:hello world
```
需要注意的是,在使用 ctypes 模块时,需要事先确定 const char* 类型变量的编码格式,如上述代码中使用的是 utf-8 编码格式。
相关问题
const char* GetDeviceMAC(unsigned int i) unsigned int GetNumberOfAttribute(const char* pDeviceMac) const char* GetAttributeName(const char* pDeviceMac, unsigned int i) python调用
在Python中调用C++函数需要使用Cython来编写包装器。以下是一个示例代码,展示了如何调用C++函数`GetDeviceMAC`、`GetNumberOfAttribute`和`GetAttributeName`:
```python
# 导入Cython模块
import cython
# 定义C++函数原型
cdef extern "C" const char* GetDeviceMAC(unsigned int)
cdef extern "C" unsigned int GetNumberOfAttribute(const char*)
cdef extern "C" const char* GetAttributeName(const char*, unsigned int)
# 包装C++函数
def get_device_mac(i: int) -> str:
return cython.cast[str](GetDeviceMAC(i))
def get_number_of_attribute(device_mac: str) -> int:
return GetNumberOfAttribute(device_mac.encode())
def get_attribute_name(device_mac: str, i: int) -> str:
return cython.cast[str](GetAttributeName(device_mac.encode(), i))
```
在上述代码中,我们使用了Cython的`cdef extern`语法来声明C++函数的原型。然后,我们定义了三个Python函数`get_device_mac`、`get_number_of_attribute`和`get_attribute_name`,这些函数分别调用了对应的C++函数,并将返回值进行适当的转换。
请注意,上述代码只是一个示例,并且假设您已经有了相应的C++库和头文件。您需要根据实际情况进行适当的修改和调试。
希望以上信息对您有所帮助!如果您有任何其他问题,请随时提问。
const char* GetAttributeName(const char* pDeviceMac, unsigned int i) python调用
要在 Python 中调用 C 函数 `GetAttributeName(const char* pDeviceMac, unsigned int i)`,你可以使用 ctypes 库来进行 C 函数的调用。首先,需要导入 ctypes 模块,并加载包含该函数的共享库。
下面是一个示例代码,演示如何调用 `GetAttributeName` 函数:
```python
import ctypes
# 加载共享库
lib = ctypes.CDLL("your_shared_library.so") # 替换为你的共享库文件名
# 定义函数参数和返回类型
lib.GetAttributeName.argtypes = [ctypes.c_char_p, ctypes.c_uint] # 参数类型为 const char* 和 unsigned int
lib.GetAttributeName.restype = ctypes.c_char_p # 返回类型为 const char*
# 调用函数
device_mac = b"device_mac" # 传递给函数的 const char* 参数,需要以字节串的形式提供
i = 0 # 传递给函数的 unsigned int 参数
attribute_name = lib.GetAttributeName(device_mac, i)
# 将返回的字符串转换为 Python 字符串
attribute_name_str = ctypes.string_at(attribute_name).decode()
# 打印属性名称
print(attribute_name_str)
```
请注意,在上面的示例中,你需要将 `"your_shared_library.so"` 替换为你实际使用的共享库的文件名。另外,根据实际情况,你可能需要调整函数的参数类型和返回类型。
这是一个基本的示例,具体的实现可能因为你所使用的共享库和操作系统而有所不同。如果你能提供更多的上下文或代码片段,我可以帮助你更具体地解决这个问题。
阅读全文
相关推荐











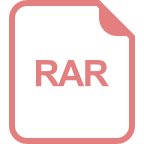




