基于python,给出人脸识别的代码
时间: 2024-10-24 10:11:19 浏览: 12
基于Python的人脸识别通常使用深度学习框架如TensorFlow、Keras或PyTorch,以及一些预训练的人脸识别模型,如FaceNet、MTCNN(Multi-task Cascaded Convolutional Networks)。下面是一个简化的例子,使用dlib库结合Haar特征检测器来进行人脸检测,以及face_recognition库进行人脸识别基础的演示:
```python
import cv2
from imutils import face_utils
import face_recognition
# 加载预加载好的人像数据库
known_faces = []
with open('known_faces.txt', 'r') as file:
known_faces_data = file.readlines()
for line in known_faces_data:
img_path, name = line.strip().split(',')
img = face_recognition.load_image_file(img_path)
encoding = face_recognition.face_encodings(img)[0]
known_faces.append((encoding, name))
# 读取视频流或摄像头捕获帧
video_capture = cv2.VideoCapture(0)
while True:
# 从摄像头获取一帧
ret, frame = video_capture.read()
if not ret:
break
# 转换为灰度图像并进行人脸检测
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = face_recognition.face_locations(gray)
for (top, right, bottom, left), name in zip(faces, known_faces):
# 提取人脸区域,并编码
face_roi = frame[top:bottom, left:right]
face_encoding = face_recognition.face_encodings(face_roi)[0]
# 检查人脸是否匹配已知人员
results = face_recognition.compare_faces([known_faces[0][0]], face_encoding)
if results[0]:
# 匹配,显示姓名
confidence = results[0] * 100
text = f"匹配:{known_faces[0][1]} ({confidence:.2f}%)"
y = top - 10 if top - 10 > 10 else top + 10
cv2.rectangle(frame, (left, y), (right, y+20), (0, 255, 0), 2)
cv2.putText(frame, text, (left, y+25), cv2.FONT_HERSHEY_SIMPLEX, 0.75, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Face Recognition", frame)
# 等待按键退出
key = cv2.waitKey(1) & 0xFF
if key == ord("q"):
break
# 释放资源
video_capture.release()
cv2.destroyAllWindows()
```
在这个示例中,你需要提前准备一个文本文件`known_faces.txt`,里面包含每个人的图片路径和名字,每行一个。注意实际应用中可能需要更复杂的人脸识别算法和性能优化。
阅读全文
相关推荐
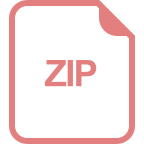
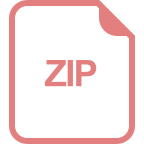
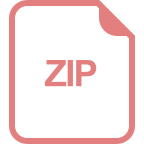
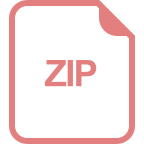
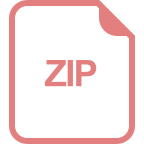
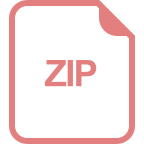
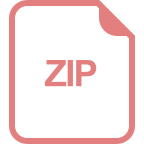
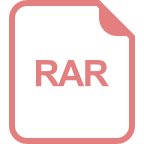
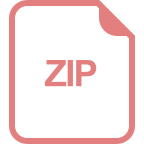
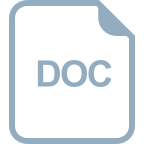
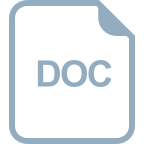
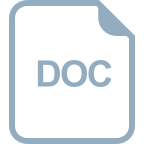
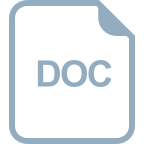



