编写基于python的人脸识别系统代码
时间: 2023-09-01 12:07:33 浏览: 52
要编写基于Python的人脸识别系统代码,您可以使用OpenCV和Dlib等Python库。下面是一些示例代码来开始:
首先,您需要安装所需的库。您可以使用以下命令在您的终端中安装它们:
```
pip install opencv-python
pip install dlib
pip install face-recognition
```
接下来,您需要导入这些库并加载您要识别的图像。以下是一个示例代码块:
```
import cv2
import dlib
import face_recognition
# 加载图片
image = cv2.imread("test.jpg")
```
接下来,您需要检测图像中的人脸。以下是一个示例代码块:
```
# 创建人脸检测器对象
detector = dlib.get_frontal_face_detector()
# 检测人脸
face_locations = detector(image, 1)
```
然后,您可以使用face_recognition库来识别人脸并为每个人脸分配标签。以下是一个示例代码块:
```
# 加载已知人脸图像
known_image = face_recognition.load_image_file("known_face.jpg")
known_face_encoding = face_recognition.face_encodings(known_image)[0]
# 为每个检测到的人脸分配标签
face_labels = []
for face_location in face_locations:
# 提取人脸编码
face_encoding = face_recognition.face_encodings(image, [face_location])[0]
# 比较人脸编码,查看是否匹配已知人脸
matches = face_recognition.compare_faces([known_face_encoding], face_encoding)
# 添加标签
if matches[0]:
face_labels.append("Known")
else:
face_labels.append("Unknown")
```
最后,您可以在图像中为每个检测到的人脸添加标签。以下是一个示例代码块:
```
# 在图像中为每个人脸添加标签
for (top, right, bottom, left), label in zip(face_locations, face_labels):
# 在人脸周围绘制矩形框
cv2.rectangle(image, (left, top), (right, bottom), (0, 255, 0), 2)
# 在人脸下面添加标签
cv2.putText(image, label, (left, bottom + 20), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 1)
```
这些是基本的示例代码块,可以帮助您开始编写基于Python的人脸识别系统代码。
相关推荐
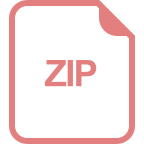
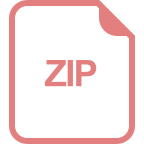
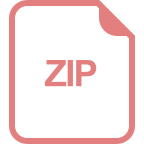














