基于python的人脸识别活体检测代码
时间: 2023-10-05 12:07:39 浏览: 122
对于人脸识别活体检测,我们需要使用深度学习框架来实现。这里我们使用Python语言和OpenCV库,以及face_recognition和dlib库来实现。
首先,我们需要安装相关的库,可以使用以下命令:
```
pip install opencv-python
pip install face_recognition
pip install dlib
```
然后我们编写代码:
```python
import cv2
import face_recognition
import dlib
# 加载人脸检测器和68个关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载模型
model = cv2.dnn.readNetFromCaffe("deploy.prototxt.txt", "res10_300x300_ssd_iter_140000.caffemodel")
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取摄像头的帧
ret, frame = cap.read()
# 缩放帧的大小,提高检测速度
small_frame = cv2.resize(frame, (0, 0), fx=0.25, fy=0.25)
# 将图像从BGR颜色空间转换为RGB颜色空间
rgb_small_frame = small_frame[:, :, ::-1]
# 检测人脸位置
faces = detector(rgb_small_frame, 0)
# 遍历每个人脸
for face in faces:
# 将人脸区域的坐标位置放大4倍
top = face.top() * 4
right = face.right() * 4
bottom = face.bottom() * 4
left = face.left() * 4
# 提取人脸区域
face_image = frame[top:bottom, left:right]
# 进行活体检测
# 将图像大小调整为300x300,并进行预处理
blob = cv2.dnn.blobFromImage(cv2.resize(face_image, (300, 300)), 1.0, (300, 300), (104.0, 177.0, 123.0))
# 将blob送入模型中进行预测
model.setInput(blob)
detections = model.forward()
# 遍历预测结果
for i in range(0, detections.shape[2]):
# 提取预测的置信度
confidence = detections[0, 0, i, 2]
# 如果置信度大于0.5,则认为是人脸
if confidence > 0.5:
# 计算人脸区域的坐标位置
box = detections[0, 0, i, 3:7] * np.array([right - left, bottom - top, right - left, bottom - top])
(startX, startY, endX, endY) = box.astype("int")
# 提取人脸区域
face_image = frame[top+startY:top+endY, left+startX:left+endX]
# 进行人脸识别
face_encoding = face_recognition.face_encodings(face_image, [(top+startY, left+startX, bottom+startY, right+startX)])[0]
# 绘制人脸区域和关键点
cv2.rectangle(frame, (left, top), (right, bottom), (0, 255, 0), 2)
landmarks = predictor(dlib.rectangle(left, top, right, bottom))
for n in range(0, 68):
x = landmarks.part(n).x
y = landmarks.part(n).y
cv2.circle(frame, (x, y), 1, (0, 0, 255), -1)
# 显示结果
cv2.imshow("Frame", frame)
cv2.waitKey(1)
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
其中,我们使用了一个预训练的深度学习模型来进行人脸检测,使用了face_recognition库来进行人脸识别,使用了dlib库来进行关键点检测。在代码中,我们还进行了活体检测,以确保识别到的人脸是真实的。最后,我们使用OpenCV库来展示识别的结果。
相关推荐
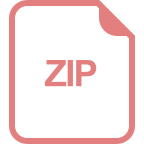














