用Django的restful framework 写一个后端进度条功能,前端用vue3
时间: 2024-03-08 12:46:04 浏览: 18
好的,下面是一个用 Django Rest Framework 和 Vue 3 实现后端进度条的示例代码:
1. 在 Django 项目的 urls.py 文件中添加以下代码:
```python
from django.urls import path
from .views import ProgressBarView
urlpatterns = [
path('progress_bar/', ProgressBarView.as_view())
]
```
2. 在 Django 项目的 views.py 文件中,编写进度条的视图函数:
```python
from rest_framework.views import APIView
from rest_framework.response import Response
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
from django.utils.decorators import method_decorator
@method_decorator(csrf_exempt, name='dispatch')
class ProgressBarView(APIView):
def post(self, request):
data = request.data
total = data.get('total')
current = data.get('current')
percentage = (current / total) * 100
response_data = {'percentage': percentage}
return JsonResponse(response_data)
```
3. 在 Vue 3 项目中,编写前端页面的代码,实现对后端进度条接口的调用和进度条的更新:
```html
<template>
<div>
<button @click="startProgress">开始进度条</button>
<div class="progress">
<div class="progress-bar" :style="{ width: progress + '%' }"></div>
</div>
</div>
</template>
<script>
import { reactive } from 'vue'
export default {
setup() {
const state = reactive({
total: 100,
current: 0,
progress: 0
})
const startProgress = () => {
const interval = setInterval(() => {
if (state.current >= state.total) {
clearInterval(interval)
} else {
state.current += 10
updateProgress()
}
}, 1000)
}
const updateProgress = () => {
fetch('/api/progress_bar/', {
method: 'POST',
body: JSON.stringify({
total: state.total,
current: state.current
}),
headers: {
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => {
state.progress = data.percentage
})
}
return {
startProgress,
state
}
}
}
</script>
<style>
.progress {
width: 100%;
height: 20px;
background-color: #f5f5f5;
border-radius: 4px;
margin-top: 10px;
}
.progress-bar {
height: 100%;
border-radius: 4px;
background-color: #007bff;
}
</style>
```
以上就是一个简单的用 Django Rest Framework 和 Vue 3 实现后端进度条的示例代码。需要注意的是,这只是一个简单的示例,实际应用中需要根据业务逻辑和前端的需求来对进度条的实现进行具体的调整。
相关推荐
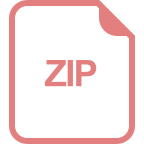
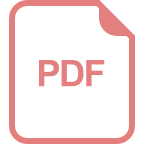
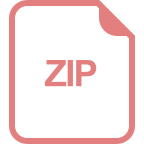














