给我评价两幅图像的ssim以及PSNR的Python代码
时间: 2024-02-19 13:59:03 浏览: 146
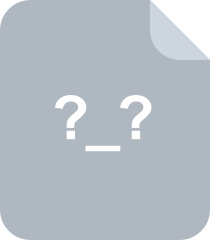
SSIM和PSNR的python代码
以下是给出两幅图像的 SSIM 和 PSNR 的 Python 代码:
```python
import cv2
import numpy as np
def ssim(image1, image2):
"""Calculate the Structural Similarity Index (SSIM) between two images."""
# Set constants
C1 = (0.01 * 255) ** 2
C2 = (0.03 * 255) ** 2
# Convert the images to grayscale
img1 = cv2.cvtColor(image1, cv2.COLOR_BGR2GRAY)
img2 = cv2.cvtColor(image2, cv2.COLOR_BGR2GRAY)
# Calculate means and variances
mu1 = cv2.GaussianBlur(img1, (11, 11), 1.5)
mu2 = cv2.GaussianBlur(img2, (11, 11), 1.5)
mu1_sq = mu1 ** 2
mu2_sq = mu2 ** 2
mu1_mu2 = mu1 * mu2
sigma1_sq = cv2.GaussianBlur(img1 ** 2, (11, 11), 1.5) - mu1_sq
sigma2_sq = cv2.GaussianBlur(img2 ** 2, (11, 11), 1.5) - mu2_sq
sigma12 = cv2.GaussianBlur(img1 * img2, (11, 11), 1.5) - mu1_mu2
# Calculate SSIM
num = (2 * mu1_mu2 + C1) * (2 * sigma12 + C2)
den = (mu1_sq + mu2_sq + C1) * (sigma1_sq + sigma2_sq + C2)
ssim = np.mean(num / den)
return ssim
def psnr(image1, image2):
"""Calculate the Peak Signal-to-Noise Ratio (PSNR) between two images."""
mse = np.mean((image1 - image2) ** 2)
if mse == 0:
return float('inf')
else:
max_pixel = 255.0
psnr = 20 * np.log10(max_pixel / np.sqrt(mse))
return psnr
```
其中,`image1` 和 `image2` 分别为两幅图像的 numpy 数组。使用方法如下:
```python
# Load the two images
image1 = cv2.imread('image1.jpg')
image2 = cv2.imread('image2.jpg')
# Calculate SSIM and PSNR
ssim_value = ssim(image1, image2)
psnr_value = psnr(image1, image2)
# Print the results
print('SSIM:', ssim_value)
print('PSNR:', psnr_value)
```
请注意,这些度量标准仅作为图像质量的参考,它们并不能完全描述图像的质量。因此,建议在实际应用中结合其他度量标准进行分析。
阅读全文
相关推荐
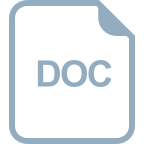
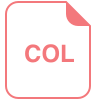
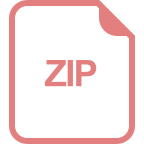
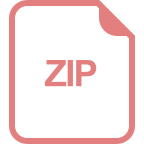
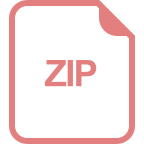
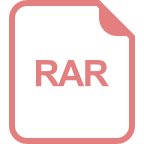
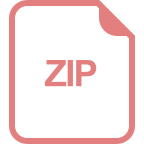
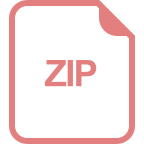
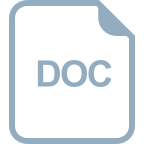
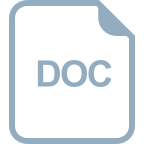





