使用c语言创建一个动态链表
时间: 2024-02-21 16:27:40 浏览: 23
好的,关于使用C语言创建一个动态链表,我们可以通过定义一个结构体来实现。代码如下:
```
#include <stdio.h>
#include <stdlib.h>
//定义链表节点结构体
typedef struct node {
int data;
struct node *next;
} Node;
//创建链表
Node* createList() {
Node *head, *tail, *pNew;
int num;
head = (Node*) malloc(sizeof(Node));
if (head == NULL) {
printf("空间不足,无法创建链表!");
exit(-1);
}
tail = head;
printf("请输入节点的值(输入-1表示链表创建完成):\n");
while (1) {
scanf("%d", &num);
if (num != -1) {
pNew = (Node*) malloc(sizeof(Node));
pNew->data = num;
tail->next = pNew;
tail = pNew;
} else {
tail->next = NULL;
break;
}
}
return head;
}
//输出链表
void printList(Node *head) {
Node *p = head->next;
printf("链表的值为:");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
Node *head, *p;
head = createList();
printList(head);
p = head;
while (p != NULL) {
Node *q = p->next;
free(p);
p = q;
}
return 0;
}
```
希望这个代码能够帮助你创建一个动态链表。如果你还有其他问题,可以随时问我哦~
相关推荐
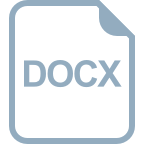
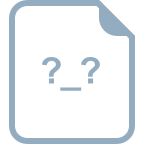
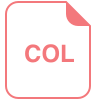
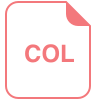
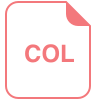
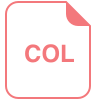
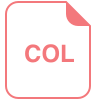









