use C program To delete a character that is the same as a character in a string, you need to use a character pointer as a function parameter. call the function in main. The function prototype is as follows: void delete_ character(char *str, char ch); [Example of input] hello,my friend! ! [Output example] hello,my friend
时间: 2024-03-18 11:39:51 浏览: 16
Sure, I can help you with that! Here's the implementation of the `delete_character` function in C:
```c
#include <stdio.h>
#include <string.h>
void delete_character(char *str, char ch) {
int i, j, len;
len = strlen(str);
for (i = 0; i < len; i++) {
if (str[i] == ch) {
for (j = i; j < len-1; j++) {
str[j] = str[j+1];
}
str[len-1] = '\0';
len--;
i--;
}
}
}
int main() {
char str[100], ch;
printf("Enter a string: ");
fgets(str, 100, stdin);
printf("Enter a character to delete: ");
scanf("%c", &ch);
delete_character(str, ch);
printf("Modified string: %s", str);
return 0;
}
```
In this code, `delete_character` function takes two parameters: a string `str` and a character `ch`. It uses two nested loops to iterate over the string and delete the characters that match the given character. The outer loop iterates over each character in the string, while the inner loop shifts the remaining characters to the left after a match is found. The function also updates the length of the string and adjusts the loop index accordingly.
In the `main` function, we take the input string and character from the user using `fgets` and `scanf` respectively. Then, we call the `delete_character` function to modify the string. Finally, we print the modified string using `printf`.
I hope this helps! Let me know if you have any questions.
相关推荐
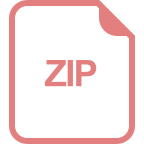
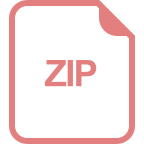
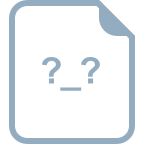
















