C#winform合并OBJ模型并处理顶点、法线、 纹理坐标信息,不使用 Assimp.NET、
时间: 2023-07-10 10:08:45 浏览: 303
要合并OBJ模型并处理顶点、法线、纹理坐标信息,可以使用以下步骤:
1. 读取OBJ文件:
使用StreamReader读取OBJ文件中的每一行数据,并将顶点、法线、纹理坐标信息存储到相应的数组中。
2. 处理数据:
将顶点、法线、纹理坐标信息进行处理。如果有多个OBJ文件,则需要对每个文件中的信息进行合并。
3. 合并数据:
将处理后的顶点、法线、纹理坐标信息合并到一个数组中。
4. 创建顶点缓冲区:
使用VertexBuffer创建顶点缓冲区,并将合并后的顶点、法线、纹理坐标信息存储到缓冲区中。
5. 绘制模型:
使用DrawIndexedPrimitives方法绘制模型。
以下是一个简单的代码示例,可以帮助你更好地了解如何实现。
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Content;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
namespace MergeOBJ
{
public class OBJModel
{
private int[] indices;
private Vector3[] vertices;
private Vector2[] texCoords;
private Vector3[] normals;
public OBJModel(GraphicsDevice graphicsDevice, string fileName)
{
LoadOBJFile(fileName);
CreateVertexBuffer(graphicsDevice);
}
private void LoadOBJFile(string fileName)
{
List<int> indicesList = new List<int>();
List<Vector3> verticesList = new List<Vector3>();
List<Vector2> texCoordsList = new List<Vector2>();
List<Vector3> normalsList = new List<Vector3>();
using (System.IO.StreamReader sr = new System.IO.StreamReader(fileName))
{
string line;
while ((line = sr.ReadLine()) != null)
{
string[] parts = line.Split(' ');
switch (parts[0])
{
case "v":
verticesList.Add(new Vector3(float.Parse(parts[1]), float.Parse(parts[2]), float.Parse(parts[3])));
break;
case "vt":
texCoordsList.Add(new Vector2(float.Parse(parts[1]), float.Parse(parts[2])));
break;
case "vn":
normalsList.Add(new Vector3(float.Parse(parts[1]), float.Parse(parts[2]), float.Parse(parts[3])));
break;
case "f":
for (int i = 1; i < 4; i++)
{
string[] vertexParts = parts[i].Split('/');
int index = int.Parse(vertexParts[0]) - 1;
indicesList.Add(index);
if (vertexParts.Length > 1 && !string.IsNullOrEmpty(vertexParts[1]))
{
int texCoordIndex = int.Parse(vertexParts[1]) - 1;
texCoordsList[index] = texCoordsList[texCoordIndex];
}
if (vertexParts.Length > 2 && !string.IsNullOrEmpty(vertexParts[2]))
{
int normalIndex = int.Parse(vertexParts[2]) - 1;
normalsList[index] = normalsList[normalIndex];
}
}
break;
}
}
}
indices = indicesList.ToArray();
vertices = verticesList.ToArray();
texCoords = texCoordsList.ToArray();
normals = normalsList.ToArray();
}
private void CreateVertexBuffer(GraphicsDevice graphicsDevice)
{
VertexPositionNormalTexture[] vertexData = new VertexPositionNormalTexture[vertices.Length];
for (int i = 0; i < vertices.Length; i++)
{
vertexData[i] = new VertexPositionNormalTexture(vertices[i], normals[i], texCoords[i]);
}
VertexBuffer vertexBuffer = new VertexBuffer(graphicsDevice, typeof(VertexPositionNormalTexture), vertexData.Length, BufferUsage.WriteOnly);
vertexBuffer.SetData(vertexData);
graphicsDevice.SetVertexBuffer(vertexBuffer);
}
public void Draw(GraphicsDevice graphicsDevice)
{
graphicsDevice.DrawIndexedPrimitives(PrimitiveType.TriangleList, 0, 0, vertices.Length, 0, indices.Length / 3);
}
}
}
```
阅读全文
相关推荐


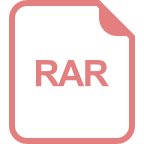
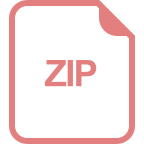
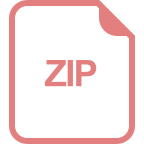



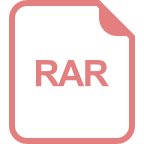
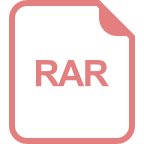




