TypeError: unsupported operand type(s) for -: 'list' and 'list'
时间: 2024-01-25 21:03:03 浏览: 166
这个错误通常出现在两个列表相减的时候,例如:
```
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = list1 - list2
```
列表是不支持减法操作的,因此会出现 TypeError。如果想要实现列表相减的操作,可以使用列表推导式或者使用 Python 的第三方库 NumPy。
使用列表推导式:
```
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = [i - j for i, j in zip(list1, list2)]
```
使用 NumPy:
```
import numpy as np
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = np.array(list1) - np.array(list2)
```
相关问题
TypeError: unsupported operand type(s) for -: 'list' and 'int'
这个错误通常是由于将一个整数从一个列表中减去而引起的。例如:
```
my_list = [1, 2, 3, 4, 5]
result = my_list - 3
```
在这个例子中,我们试图从列表 `my_list` 中减去一个整数 `3`,这是不允许的。要解决这个错误,我们需要确定哪个变量是列表,然后改变操作使其符合列表的要求。例如,如果我们想从列表中减去一个数字,我们可以使用列表推导式或循环来实现:
```
my_list = [1, 2, 3, 4, 5]
result = [num - 3 for num in my_list]
```
在这个例子中,我们使用列表推导式来创建一个新的列表,其中每个元素都从原始列表中减去了 `3`。现在 `result` 将是 `[-2, -1, 0, 1, 2]`。
如果您能提供更多的代码和上下文,我可以给出更具体的解决方案。
TypeError: unsupported operand type(s) for -: 'list' and 'tuple'
This error occurs when you try to subtract a tuple from a list or vice versa.
For example:
```
list1 = [1, 2, 3]
tuple1 = (4, 5, 6)
result = list1 - tuple1
```
This will raise a TypeError because you cannot subtract a tuple from a list.
To avoid this error, make sure you are performing operations between objects of the same type. If you need to convert one type to another, use the appropriate conversion function. For example, you can convert a list to a tuple using the `tuple()` function:
```
list1 = [1, 2, 3]
tuple1 = (4, 5, 6)
result = tuple(list1) - tuple1
```
阅读全文
相关推荐
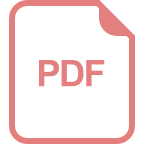
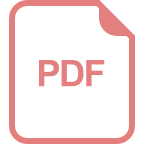







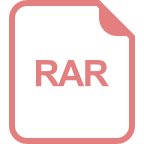
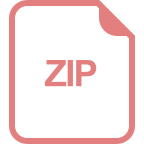
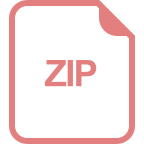
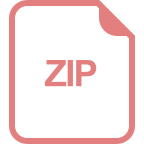
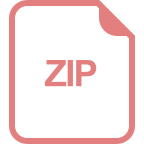