用java写一段工厂模式
时间: 2023-10-18 19:06:50 浏览: 28
好的,这个问题很适合我回答。下面是用Java编写的工厂模式示例代码:
```java
public interface Shape {
void draw();
}
public class Rectangle implements Shape {
public void draw() {
System.out.println("Inside Rectangle::draw() method.");
}
}
public class Square implements Shape {
public void draw() {
System.out.println("Inside Square::draw() method.");
}
}
public class Circle implements Shape {
public void draw() {
System.out.println("Inside Circle::draw() method.");
}
}
public class ShapeFactory {
public Shape getShape(String shapeType){
if(shapeType == null){
return null;
}
if(shapeType.equalsIgnoreCase("CIRCLE")){
return new Circle();
} else if(shapeType.equalsIgnoreCase("RECTANGLE")){
return new Rectangle();
} else if(shapeType.equalsIgnoreCase("SQUARE")){
return new Square();
}
return null;
}
}
public class FactoryPatternDemo {
public static void main(String[] args) {
ShapeFactory shapeFactory = new ShapeFactory();
Shape shape1 = shapeFactory.getShape("CIRCLE");
Shape shape2 = shapeFactory.getShape("RECTANGLE");
Shape shape3 = shapeFactory.getShape("SQUARE");
shape1.draw();
shape2.draw();
shape3.draw();
}
}
```
在这个工厂模式示例中,我们定义了一个Shape接口和三个实现类。我们还定义了一个ShapeFactory类,它根据客户端传递的字符串参数来生成相应的对象。在FactoryPatternDemo类中,我们使用ShapeFactory来获取Shape对象,并调用它们的draw方法。
相关推荐
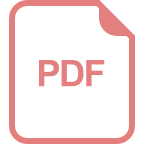
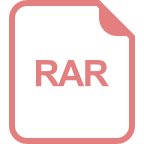
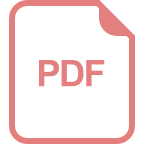














