安卓,写一个程序,要求:用fragment写,上面是textview,下面一个fragment里有3个按钮,为按钮设置监听器,点击按钮上面切换显示不同的布局
时间: 2023-06-24 09:04:42 浏览: 154
好的,以下是一个简单的示例代码:
首先,我们需要创建一个包含TextView和Fragment的Activity:
```java
public class MainActivity extends AppCompatActivity {
private TextView textView;
private ButtonFragment buttonFragment;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化TextView
textView = findViewById(R.id.text_view);
// 初始化ButtonFragment
buttonFragment = new ButtonFragment();
getSupportFragmentManager().beginTransaction()
.replace(R.id.button_fragment_container, buttonFragment)
.commit();
}
// 切换布局
public void switchLayout(int layoutId) {
getSupportFragmentManager().beginTransaction()
.replace(R.id.fragment_container, new LayoutFragment(layoutId))
.commit();
}
}
```
在Activity中,我们初始化了一个TextView和一个ButtonFragment,并将ButtonFragment添加到布局中。还定义了一个名为`switchLayout`的方法,用于切换显示不同的布局。
接下来,我们需要创建一个ButtonFragment,包含3个按钮:
```java
public class ButtonFragment extends Fragment implements View.OnClickListener {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_button, container, false);
// 初始化按钮和设置监听器
view.findViewById(R.id.button1).setOnClickListener(this);
view.findViewById(R.id.button2).setOnClickListener(this);
view.findViewById(R.id.button3).setOnClickListener(this);
return view;
}
@Override
public void onClick(View v) {
// 获取MainActivity实例并调用switchLayout方法切换布局
MainActivity activity = (MainActivity) getActivity();
switch (v.getId()) {
case R.id.button1:
activity.switchLayout(R.layout.layout1);
break;
case R.id.button2:
activity.switchLayout(R.layout.layout2);
break;
case R.id.button3:
activity.switchLayout(R.layout.layout3);
break;
}
}
}
```
ButtonFragment中主要是初始化3个按钮并设置它们的监听器。在点击按钮时,调用MainActivity中的`switchLayout`方法,并传入不同布局的id值。
最后,我们需要创建一个LayoutFragment,用于显示不同的布局:
```java
public class LayoutFragment extends Fragment {
private int layoutId;
public LayoutFragment(int layoutId) {
this.layoutId = layoutId;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(layoutId, container, false);
}
}
```
LayoutFragment的构造函数接收一个布局id值,然后在`onCreateView`方法中将该布局加载并返回。
最后,我们还需要创建以下4个布局文件:
activity_main.xml:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="This is the main layout"
android:textSize="20sp" />
<FrameLayout
android:id="@+id/button_fragment_container"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/text_view" />
<FrameLayout
android:id="@+id/fragment_container"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/button_fragment_container" />
</RelativeLayout>
```
fragment_button.xml:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Layout 1" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Layout 2" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Layout 3" />
</LinearLayout>
```
layout1.xml:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal|center_vertical"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is layout 1"
android:textSize="20sp" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_launcher_background" />
</LinearLayout>
```
layout2.xml:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is layout 2"
android:textSize="20sp"
android:layout_centerInParent="true" />
<Button
android:id="@+id/layout2_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click me!"
android:layout_below="@id/text_view"
android:layout_centerHorizontal="true" />
</RelativeLayout>
```
layout3.xml:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal|center_vertical"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is layout 3"
android:textSize="20sp" />
<Button
android:id="@+id/layout3_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click me!" />
</LinearLayout>
```
以上就是一个简单的示例,通过点击ButtonFragment中的按钮,可以切换显示不同的布局。希望能对你有所帮助!
阅读全文
相关推荐
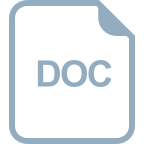
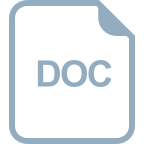
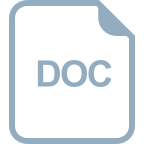












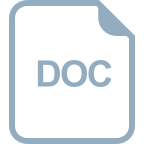
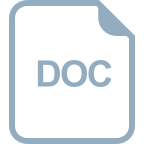
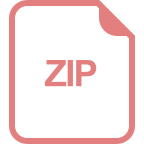
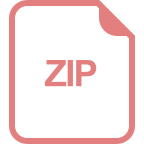