Python爬虫爬取腾讯新闻代码
时间: 2023-11-21 20:40:45 浏览: 125
以下是一个简单的 Python 爬虫,可以爬取腾讯新闻的标题和链接:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://news.qq.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
news_list = soup.find_all('a', {'class': 'linkto'})
for news in news_list:
print(news.get_text())
print(news['href'])
```
首先,我们需要导入 `requests` 和 `BeautifulSoup` 库。然后,我们定义了要爬取的腾讯新闻网址,并使用 `requests` 库获取网页的内容。接下来,我们使用 `BeautifulSoup` 库解析 HTML 并保存在 `soup` 变量中。
然后,我们使用 `find_all` 方法查找所有具有 `linkto` 类的链接标签,并将它们保存在 `news_list` 列表中。最后,我们遍历该列表并打印每个新闻的标题和链接。
当然,这只是一个简单的示例,你可以根据自己的需求修改代码以获取更多的内容。另外,要注意不要频繁地发送请求,以避免被封 IP。
相关问题
python爬虫爬取腾讯vip
Python爬虫是一种用于自动化获取网数据的技术,可以用来爬取腾讯VIP的内容。下面是一个简单的Python爬虫爬取腾讯VIP的示例代码:
```python
import requests
# 发送HTTP请求获取网页内容
url = "https://v.qq.com/"
response = requests.get(url)
html = response.text
# 解析网页内容,提取腾讯VIP相关信息
# 这里可以使用第三方库,如BeautifulSoup或正则表达式等进行解析
# 处理提取到的数据,如保存到本地文件或进行进一步处理
```
上述代码使用了Python的requests库发送HTTP请求获取网页内容,并使用response.text获取到网页的HTML源码。接下来可以使用第三方库(如BeautifulSoup或正则表达式等)对HTML源码进行解析,提取出腾讯VIP相关的信息。最后可以根据需求对提取到的数据进行处理,如保存到本地文件或进行进一步处理。
python爬虫爬取腾讯招聘
您好!关于爬取腾讯招聘信息的问题,您可以使用Python爬虫库requests和BeautifulSoup来实现。以下是一个简单的示例代码,可以爬取腾讯招聘网站上的职位信息:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://careers.tencent.com/search.html?index=%d&keyword=python'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
for i in range(1, 5):
response = requests.get(url % i, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
items = soup.select('.recruit-list .recruit-item')
for item in items:
title = item.select_one('.recruit-title').text.strip()
location = item.select_one('.recruit-location').text.strip()
category = item.select_one('.recruit-category').text.strip()
print(title, location, category)
```
以上代码通过循环爬取了腾讯招聘网站上前4页的职位信息,并输出了职位名称、工作地点和职位类别。您也可以根据自己的需求进行修改。
阅读全文
相关推荐
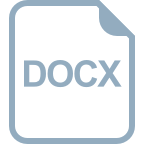
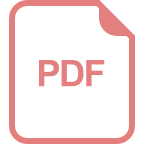
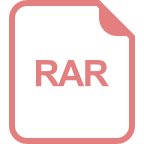













