怎么用jsp和servlet获得含有用户名 varchar 性别enum("1""2")和time datetime的预约信息表的信息,具体代码和文件
时间: 2024-03-04 19:49:00 浏览: 46
首先,你需要在数据库中创建一个名为“appointment”的表,该表应包含以下列:
- id:int类型,主键,自动递增
- username:varchar类型,表示预约者的用户名
- gender:enum类型,表示预约者的性别
- time:datetime类型,表示预约时间
接下来,你可以使用以下代码来编写一个Servlet,以获取预约信息表中的信息:
```java
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/appointment")
public class AppointmentServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private Connection conn;
public void init() throws ServletException {
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/mydb";
String username = "root";
String password = "password";
conn = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
List<Appointment> appointments = new ArrayList<Appointment>();
try {
PreparedStatement ps = conn.prepareStatement("SELECT * FROM appointment");
ResultSet rs = ps.executeQuery();
while (rs.next()) {
Appointment appointment = new Appointment();
appointment.setId(rs.getInt("id"));
appointment.setUsername(rs.getString("username"));
appointment.setGender(rs.getString("gender"));
appointment.setTime(rs.getString("time"));
appointments.add(appointment);
}
rs.close();
ps.close();
} catch (SQLException e) {
e.printStackTrace();
}
request.setAttribute("appointments", appointments);
request.getRequestDispatcher("/appointments.jsp").forward(request, response);
}
public void destroy() {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在本例中,我们使用MySQL数据库。如果你使用的是其他数据库,你需要更改驱动程序的名称和连接URL。
在JSP文件中,你可以使用以下代码来显示预约信息表中的信息:
```html
<% List<Appointment> appointments = (List<Appointment>) request.getAttribute("appointments"); %>
<table>
<thead>
<tr>
<th>ID</th>
<th>Username</th>
<th>Gender</th>
<th>Time</th>
</tr>
</thead>
<tbody>
<% for (Appointment appointment : appointments) { %>
<tr>
<td><%= appointment.getId() %></td>
<td><%= appointment.getUsername() %></td>
<td><%= appointment.getGender() %></td>
<td><%= appointment.getTime() %></td>
</tr>
<% } %>
</tbody>
</table>
```
最后,你需要在web.xml文件中使用以下代码来映射Servlet:
```xml
<servlet>
<servlet-name>AppointmentServlet</servlet-name>
<servlet-class>com.example.AppointmentServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>AppointmentServlet</servlet-name>
<url-pattern>/appointment</url-pattern>
</servlet-mapping>
```
请注意,你需要将这些代码添加到你的项目中,并将其配置为适合你的项目。
阅读全文
相关推荐


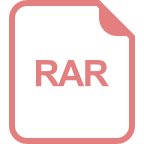
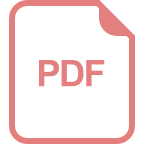
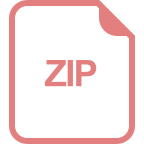
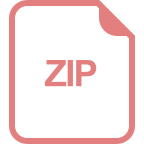
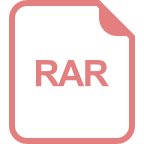
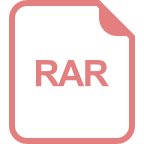
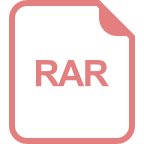
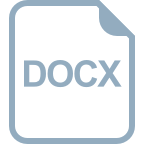
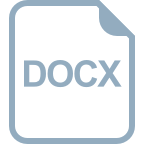
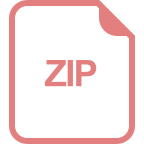
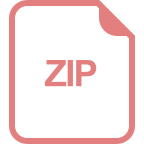
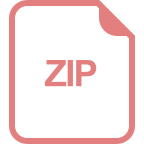
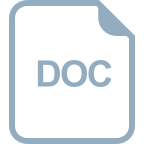
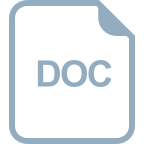