C++ protobuf base64
时间: 2023-11-09 21:09:44 浏览: 115
C++ protobuf是Google开发的一种高效的序列化数据格式,可以用于不同语言之间的数据交换。它可以将结构化数据序列化为二进制格式,以便在网络上传输或在磁盘上存储。而base64是一种编码方式,可以将二进制数据转换为可打印的ASCII字符,以便在文本协议中传输。在使用protobuf通过websocket发送图像时,可以将图像数据先序列化为protobuf格式,然后再将其转换为base64编码的字符串,最后通过websocket发送给接收方。在C++中,可以使用protobuf提供的--cpp_out选项生成相应的C++代码,以便在程序中使用protobuf进行数据序列化和反序列化。
相关问题
protobuf base64编码, cpp列子
以下是一个简单的 C++ 列子,展示了如何使用 protobuf 库进行 base64 编码:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <fstream>
#include <google/protobuf/message.h>
#include <google/protobuf/io/zero_copy_stream_impl_lite.h>
#include <google/protobuf/io/coded_stream.h>
#include <google/protobuf/util/base64.h>
using namespace std;
using namespace google::protobuf;
using namespace google::protobuf::io;
using namespace google::protobuf::util;
// 将 protobuf 消息进行 base64 编码
string protobufToBase64(const Message& message) {
string result;
string messageString;
// 将 protobuf 消息序列化为字符串
message.SerializeToString(&messageString);
// 对序列化后的字符串进行编码
Base64Encode(messageString, &result);
return result;
}
// 从 base64 编码中解码出 protobuf 消息
bool base64ToProtobuf(const string& base64String, Message& message) {
string decoded;
if (!Base64Decode(base64String, &decoded)) {
return false;
}
// 使用 ZeroCopyInputStream 和 CodedInputStream 解码出消息
const char* buffer = decoded.c_str();
int size = decoded.size();
ZeroCopyInputStream* input = new ArrayInputStream(buffer, size);
CodedInputStream* codedInput = new CodedInputStream(input);
codedInput->SetTotalBytesLimit(size, size);
bool success = message.ParseFromCodedStream(codedInput);
delete codedInput;
delete input;
return success;
}
int main() {
// 假设我们有一个 protobuf 消息 MyMessage,需要进行编码
MyMessage message;
message.set_id(123);
message.set_name("Hello World");
string encoded = protobufToBase64(message);
cout << "Encoded: " << encoded << endl;
// 假设我们已经从某个地方得到了 base64 编码,需要解码成 protobuf 消息
MyMessage decodedMessage;
bool success = base64ToProtobuf(encoded, decodedMessage);
if (success) {
cout << "Decoded ID: " << decodedMessage.id() << endl;
cout << "Decoded Name: " << decodedMessage.name() << endl;
} else {
cout << "Decoding failed." << endl;
}
return 0;
}
```
在该例子中,我们使用了 `google::protobuf::util::Base64Encode()` 和 `google::protobuf::util::Base64Decode()` 函数进行 base64 编码和解码。为了从 base64 编码中解码出 protobuf 消息,我们首先需要使用 `google::protobuf::util::Base64Decode()` 函数将 base64 编码解码成二进制数据,然后再使用 `google::protobuf::Message::ParseFromCodedStream()` 函数将二进制数据解析成 protobuf 消息。
pb 解析base64 图片
PB(Protocol Buffers),是一种由Google开发的序列化数据格式,用于在各种平台之间高效地传输结构化的数据。当你需要解析Base64编码的图片时,首先你需要将Base64字符串解码成字节流,然后读取这些字节并按照PB协议的规则解析。
以下是基本步骤:
1. **解码Base64**:使用相应的库(如Python的`base64`模块,JavaScript的`atob`函数等)将Base64字符串转换回原始二进制数据。
2. **创建PB对象**:如果你有一个预先定义好的PB消息结构(`.proto`文件),对应于图像的数据类型,比如`Image`或`ByteString`,你可以使用这个结构来解析数据。
3. **初始化PB实例**:根据消息类型,实例化一个`Image`对象或者其他适合的PB类型。
4. **设置字段值**:将解码后的二进制数据赋给该对象的`data`或其他表示图像数据的字段。
5. **验证和解析**:使用PB库提供的方法,如`ParseFromString`(Python)或`parseFromBytes`(C++),解析整个字节数组到PB对象中。
```python
import base64
from google.protobuf import any_pb2
def parse_base64_to_pb(base64_str, image_type):
decoded_data = base64.b64decode(base64_str)
image_message = any_pb2.Any()
image_message.Pack(image_type, decoded_data) # 将解码后的数据包装到Any对象中
return image_message
# 使用示例
image_proto = parse_base64_to_pb(your_base64_string, your_image_type)
```
阅读全文
相关推荐
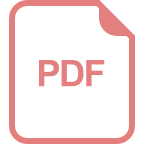
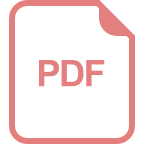
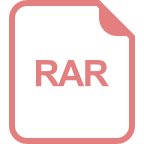
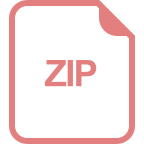
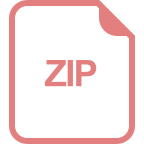
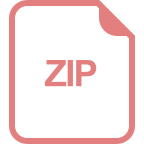
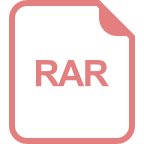
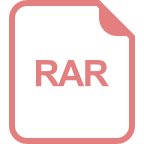
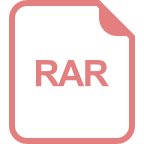
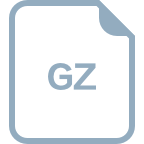
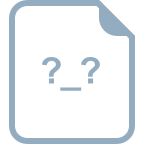
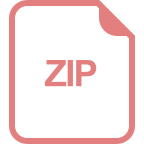
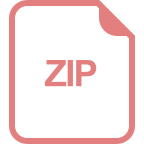
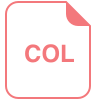

