随机数扑克检测源代码
时间: 2023-07-01 13:12:29 浏览: 225
以下是一份使用C++编写的随机数扑克检测的源代码:
```
#include <iostream>
#include <cmath>
#include <ctime>
#include <cstdlib>
using namespace std;
// 定义扑克牌的花色和点数
const int SPADES = 0;
const int HEARTS = 1;
const int CLUBS = 2;
const int DIAMONDS = 3;
const int JACK = 11;
const int QUEEN = 12;
const int KING = 13;
const int ACE = 14;
// 定义扑克牌的结构体
struct Card {
int rank;
int suit;
};
// 随机生成一张扑克牌
Card getRandomCard() {
Card card;
card.rank = rand() % 13 + 2;
card.suit = rand() % 4;
return card;
}
// 检测扑克牌是否符合随机性
bool isRandom(Card cards[], int n) {
int counts[15][4] = {0};
for (int i = 0; i < n; i++) {
counts[cards[i].rank][cards[i].suit]++;
}
for (int i = 2; i <= 14; i++) {
for (int j = 0; j < 4; j++) {
if (counts[i][j] > 1) {
return false;
}
}
}
return true;
}
int main() {
srand(time(NULL));
int n = 10000;
Card cards[n];
for (int i = 0; i < n; i++) {
cards[i] = getRandomCard();
}
if (isRandom(cards, n)) {
cout << "The cards are random." << endl;
} else {
cout << "The cards are not random." << endl;
}
return 0;
}
```
该源代码首先定义了扑克牌的花色和点数,然后定义了一个结构体Card用于表示一张扑克牌。接着,定义了函数getRandomCard用于随机生成一张扑克牌,函数isRandom用于检测扑克牌是否符合随机性。最后,在主函数中随机生成一组扑克牌,并通过isRandom函数检测其随机性。
阅读全文
相关推荐
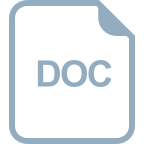
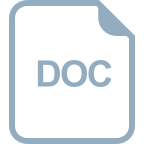
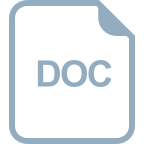
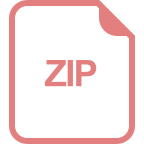
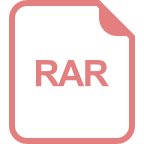
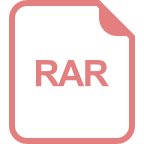
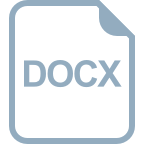
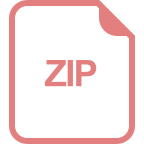
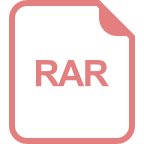
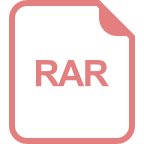
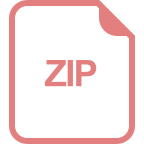
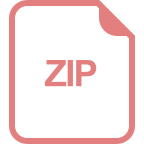
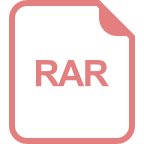
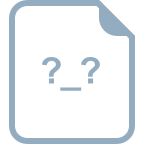
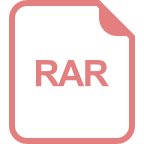
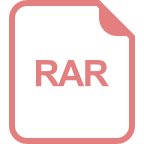
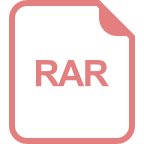
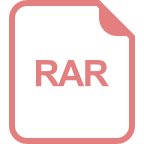
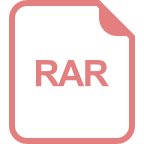