用c++给出一个windows所有文件读写模式的例子
时间: 2024-05-16 16:14:24 浏览: 14
以下是一个简单的示例程序,演示了如何使用 C++ 在 Windows 中打开、读取和写入文件,以及设置不同的文件读写模式:
```cpp
#include <iostream>
#include <fstream>
#include <windows.h>
int main() {
// 打开文件
HANDLE hFile = CreateFile("test.txt", GENERIC_READ | GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (hFile == INVALID_HANDLE_VALUE) {
std::cerr << "Failed to open file!" << std::endl;
return 1;
}
// 写入数据
const char* data = "Hello, world!";
DWORD numBytesWritten;
if (!WriteFile(hFile, data, strlen(data), &numBytesWritten, NULL)) {
std::cerr << "Failed to write to file!" << std::endl;
CloseHandle(hFile);
return 1;
}
// 读取数据
char buffer[1024];
DWORD numBytesRead;
if (!ReadFile(hFile, buffer, sizeof(buffer), &numBytesRead, NULL)) {
std::cerr << "Failed to read from file!" << std::endl;
CloseHandle(hFile);
return 1;
}
// 输出数据
std::cout << "File contents: " << buffer << std::endl;
// 关闭文件
CloseHandle(hFile);
return 0;
}
```
在上面的示例中,我们使用 `CreateFile` 函数打开了一个名为 "test.txt" 的文件,并设置了 `GENERIC_READ` 和 `GENERIC_WRITE` 标志来指定我们想要读取和写入文件。我们还指定了 `CREATE_ALWAYS` 标志来表示如果文件不存在,则创建它;如果文件已经存在,则截断文件并将其大小设置为 0。
我们使用 `WriteFile` 函数向文件中写入了一些数据,然后使用 `ReadFile` 函数从文件中读取数据。最后,我们将读取的数据输出到控制台,并使用 `CloseHandle` 函数关闭文件句柄。
请注意,上面的示例中使用的是默认的文件读写模式,即非重叠模式。如果您需要使用重叠模式,您需要使用 `CreateFile` 函数的 `FILE_FLAG_OVERLAPPED` 标志。
相关推荐
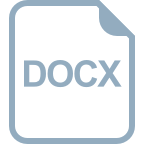
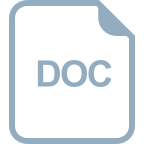
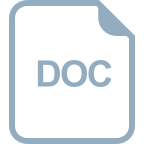














