帮我生成一段使用C#的将多组name和value导入到xml,并且可读取xml的name和value的代码
时间: 2024-03-09 17:44:41 浏览: 89
好的,以下是一段使用C#将多组name和value导入到xml,并且可读取xml的name和value的代码:
```csharp
using System;
using System.Xml;
class Program
{
static void Main(string[] args)
{
// 创建一个 XmlDocument 对象
XmlDocument xmlDoc = new XmlDocument();
// 创建根节点
XmlElement root = xmlDoc.CreateElement("root");
xmlDoc.AppendChild(root);
// 创建多组 name 和 value
string[] names = { "name1", "name2", "name3" };
string[] values = { "value1", "value2", "value3" };
// 循环创建子节点
for (int i = 0; i < names.Length; i++)
{
// 创建子节点
XmlElement child = xmlDoc.CreateElement("child");
root.AppendChild(child);
// 创建 name 和 value 属性
XmlAttribute nameAttr = xmlDoc.CreateAttribute("name");
nameAttr.Value = names[i];
child.Attributes.Append(nameAttr);
XmlAttribute valueAttr = xmlDoc.CreateAttribute("value");
valueAttr.Value = values[i];
child.Attributes.Append(valueAttr);
}
// 保存文档
xmlDoc.Save("data.xml");
// 读取文档
xmlDoc.Load("data.xml");
// 获取根节点
root = xmlDoc.DocumentElement;
// 遍历子节点
foreach (XmlElement child in root.ChildNodes)
{
// 获取 name 和 value 属性
string name = child.Attributes["name"].Value;
string value = child.Attributes["value"].Value;
// 输出结果
Console.WriteLine("{0}: {1}", name, value);
}
}
}
```
这段代码首先创建一个 XmlDocument 对象,并创建一个名为 "root" 的根节点。然后,使用循环创建多个子节点,并在每个子节点上添加 name 和 value 属性。最后,将文档保存到文件中,并重新加载文档以读取数据。
在读取数据时,我们可以使用 DocumentElement 属性获取根节点,然后使用 ChildNodes 属性遍历所有子节点,并从每个子节点的 Attributes 集合中获取 name 和 value 属性的值。
阅读全文
相关推荐
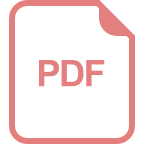
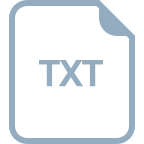
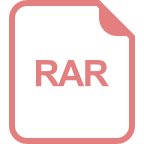
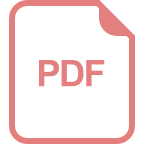
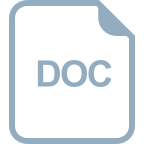
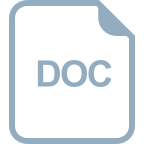
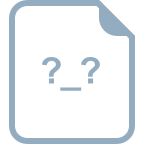
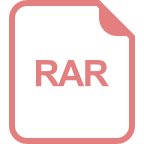
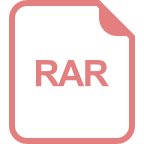
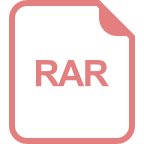
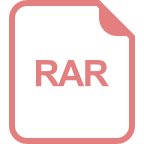
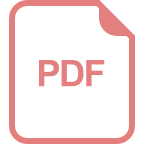
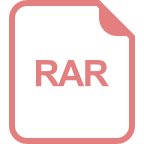
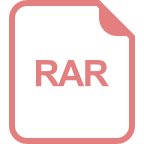
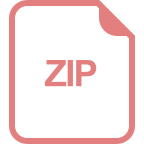
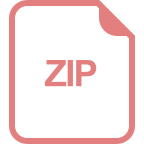

