c语言编写FM1208cpu卡发卡读卡具体代码
时间: 2023-08-12 11:30:58 浏览: 260
以下是一个简单的使用C语言编写的FM1208 CPU卡发卡和读卡的代码示例,其中使用了串口通信和APDU指令:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <termios.h>
#include <fcntl.h>
#include <unistd.h>
#define MAX_BUF_LEN 1024
int serialOpen(char *port, int baudrate)
{
int fd;
struct termios newtio;
fd = open(port, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0)
{
perror("open");
return -1;
}
tcgetattr(fd, &newtio);
memset(&newtio, 0, sizeof(newtio));
newtio.c_cflag = baudrate | CS8 | CLOCAL | CREAD;
newtio.c_iflag = IGNPAR;
newtio.c_oflag = 0;
newtio.c_lflag = 0;
newtio.c_cc[VTIME] = 0;
newtio.c_cc[VMIN] = 1;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &newtio);
return fd;
}
void serialClose(int fd)
{
close(fd);
}
int sendAPDU(int fd, unsigned char *apdu, int apduLen, unsigned char *respBuf, int *respLen)
{
unsigned char buf[MAX_BUF_LEN];
int len;
write(fd, apdu, apduLen);
len = read(fd, buf, MAX_BUF_LEN);
memcpy(respBuf, buf, len);
*respLen = len;
return 0;
}
int main(int argc, char **argv)
{
int fd;
unsigned char apdu[256] = {0};
unsigned char respBuf[MAX_BUF_LEN] = {0};
int respLen = 0;
// 打开串口
fd = serialOpen("/dev/ttyS0", B9600);
if (fd < 0)
{
printf("open serial port failed\n");
return -1;
}
// 发送APDU指令
// 发卡
apdu[0] = 0x00;
apdu[1] = 0xA4;
apdu[2] = 0x04;
apdu[3] = 0x00;
apdu[4] = 0x08;
apdu[5] = 0xA0;
apdu[6] = 0x00;
apdu[7] = 0x00;
apdu[8] = 0x00;
apdu[9] = 0x03;
apdu[10] = 0xF0;
apdu[11] = 0x01;
apdu[12] = 0x01;
apdu[13] = 0x05;
sendAPDU(fd, apdu, 14, respBuf, &respLen);
memset(apdu, 0, sizeof(apdu));
memset(respBuf, 0, sizeof(respBuf));
respLen = 0;
// 读卡
apdu[0] = 0x00;
apdu[1] = 0xB0;
apdu[2] = 0x00;
apdu[3] = 0x00;
apdu[4] = 0x08;
sendAPDU(fd, apdu, 5, respBuf, &respLen);
// 处理卡片响应
if (respBuf[respLen - 2] == 0x90 && respBuf[respLen - 1] == 0x00)
{
printf("Read card success\n");
}
else
{
printf("Read card failed\n");
}
// 关闭串口
serialClose(fd);
return 0;
}
```
需要注意的是,上述代码仅供参考,具体的代码实现需要根据所使用的硬件平台和应用场景进行相应的调整和修改。
阅读全文
相关推荐
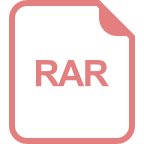
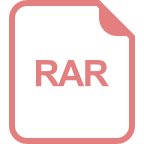
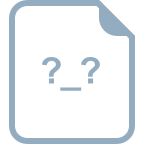
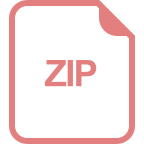
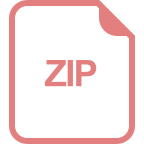
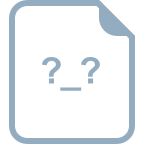