写一篇c++简易学生信息管理系统的代码
时间: 2024-11-18 14:12:34 浏览: 0
在C++中编写一个简单的学生信息系统管理系统,我们可以创建一个包含基本属性如姓名、学号和成绩的结构体,然后使用类来操作这个结构体。下面是一个基本的实现示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义学生信息结构体
struct Student {
string name;
int id;
double score;
};
// 学生管理类
class StudentManagement {
private:
vector<Student> students; // 使用向量存储学生列表
public:
// 添加学生
void addStudent(const string& name, int id, double score) {
Student newStudent = {name, id, score};
students.push_back(newStudent);
}
// 删除学生
void removeStudent(int id) {
for (auto it = students.begin(); it != students.end(); ++it) {
if (it->id == id) {
students.erase(it);
break;
}
}
}
// 修改学生信息
void updateStudent(int id, const string& newName, double newScore) {
for (auto& student : students) {
if (student.id == id) {
student.name = newName;
student.score = newScore;
return;
}
}
}
// 查找学生
void searchStudent(int id) {
for (const auto& student : students) {
if (student.id == id) {
cout << "Name: " << student.name << ", ID: " << student.id << ", Score: " << student.score << endl;
return;
}
}
cout << "Student not found." << endl;
}
};
int main() {
StudentManagement system;
// 示例操作
system.addStudent("张三", 001, 85.0);
system.addStudent("李四", 002, 90.0);
system.searchStudent(001); // 查找张三的信息
system.updateStudent(001, "王五", 92.0); // 更新张三的姓名和成绩
system.removeStudent(002); // 删除李四
return 0;
}
```
阅读全文
相关推荐
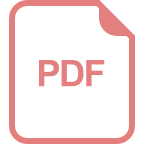
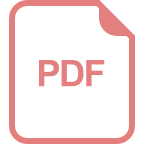
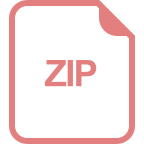
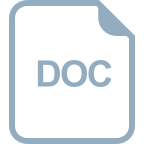
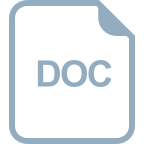
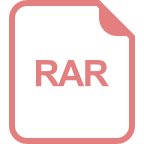
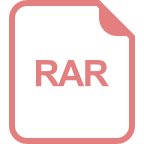
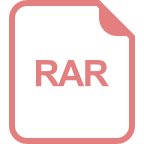
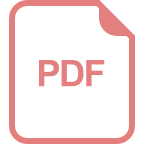
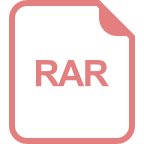
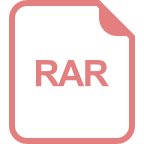
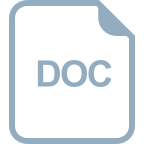
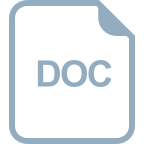
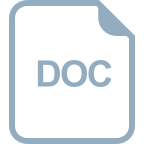
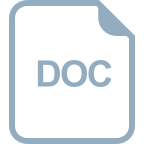
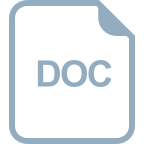
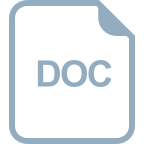
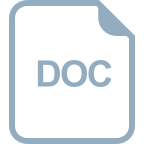
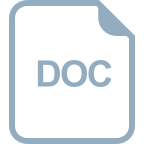