输入某年某月某日,判断这一天是这一年的第几天?程序分析:以3月5日为例,应该先把前两个月的加起来,然后再加上5天即本年的第几天,特殊情况,闰年且输入月份大于3时需考虑多加一天。\n**输入格式要求:\"%
时间: 2023-05-31 21:18:23 浏览: 130
### 回答1:
Y年M月D日\",例如:\"2022年3月5日\"。**
可以按照以下步骤编写程序:
1. 从输入中获取年、月、日的值。
2. 根据输入的年份判断是否为闰年,如果是闰年且输入月份大于2月,则本年的天数需要加1。
3. 创建一个列表,存储每个月份的天数。
4. 遍历列表,将输入月份之前的所有月份的天数相加,再加上输入的日数,即为这一天是这一年的第几天。
代码如下:
```python
date = input("请输入日期,格式为\"YYYY年MM月DD日\":")
year = int(date[:4])
month = int(date[5:7])
day = int(date[8:10])
if (year % 4 == and year % 100 != ) or year % 400 == :
if month > 2:
days_of_year = 366
else:
days_of_year = 365
else:
days_of_year = 365
days_of_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if (year % 4 == and year % 100 != ) or year % 400 == :
days_of_month[1] = 29
days = sum(days_of_month[:month-1]) + day
print("{}是{}年的第{}天。".format(date, year, days))
```
示例输出:
```
请输入日期,格式为"YYYY年MM月DD日":2022年3月5日
2022年3月5日是2022年的第64天。
```
### 回答2:
这个问题涉及到一个简单的日期计算问题。我们可以通过程序算出来某一天是这一年的第几天。先看一下程序的分析,以3月5日为例:
- 该日期前面两个月的天数相加,即1月份和2月份总共多少天
- 再加上该日期的天数,即5天,得到天数总和
- 判断这一年是否为闰年,如果是闰年且输入月份大于3,则总天数需要再加1天
基于上面的分析,我们可以写出下面的Python程序:
```
import datetime
date_str = input("请输入日期(格式:yyyy-mm-dd):")
year, month, day = map(int, date_str.split("-"))
today = datetime.date(year, month, day)
beginning = datetime.date(year, 1, 1)
delta = today - beginning + datetime.timedelta(days=1)
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
if month > 2:
delta += datetime.timedelta(days=1)
print("{0}年{1}月{2}日是{0}年的第{3}天。".format(year, month, day, delta.days))
```
上面的程序使用了Python标准库中的datetime模块来处理日期。首先输入一个日期,通过字符串分割得到年、月、日三个数字.然后使用datetime.date来分别构造出今天和今年第一天的日期对象,然后使用减法运算得到相差的天数。最后加上判断闰年和计算2月29日的代码,输出计算结果。
在输出结果时,使用字符串的format方法,将年、月、日和相差的天数组成一个字符串输出。
在实际的开发工作中,我们可能会遇到更复杂的日期计算问题。这时候需要灵活掌握日期处理的技巧和相关的编程库知识,才能解决这些问题。
### 回答3:
本题需要用到日期计算,主要有两个要点,第一是要判断是否为闰年,第二是要将输入的月份之前的天数加起来再加上输入的日期。下面就从这两点来讲解。
一、判断是否为闰年
在公元年份可被4整除的条件下,将该年份标记为闰年。但如果这个年份可以被100整除,且又不被400整除的话,则不是闰年。例如1900年就是这种情况,不能被4整除,因此不是闰年;而2000年被400整除,因此是闰年。
这个判断过程可以用下面的代码实现:
bool isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return true; // 是闰年
} else {
return false; // 不是闰年
}
}
其中%是求余运算符,即a%b的结果为a除以b的余数。
二、将输入的月份之前的天数加起来再加上输入的日期
这个过程需要我们提前定义一个数组,表示每个月份的天数,代码如下:
int days[12] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
其中days[0]表示1月份的天数,以此类推。
有了这个数组,我们就可以根据输入的日期计算出这一天是这一年的第几天。先将输入的月份之前的天数加起来:
int month, day;
cin >> year >> month >> day;
int result = 0;
for (int i = 0; i < month - 1; ++i) {
result += days[i];
}
然后再加上输入的日期:
result += day;
如果这一年是闰年,且输入的月份大于2,则需要再加上1:
if (isLeapYear(year) && month > 2) {
result += 1;
}
最后输出结果即可:
cout << result << endl;
完整代码如下:
#include <iostream>
using namespace std;
bool isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return true; // 是闰年
} else {
return false; // 不是闰年
}
}
int main() {
int year, month, day;
int days[12] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
cin >> year >> month >> day;
int result = 0;
for (int i = 0; i < month - 1; ++i) {
result += days[i];
}
result += day;
if (isLeapYear(year) && month > 2) {
result += 1;
}
cout << result << endl;
return 0;
}
相关推荐
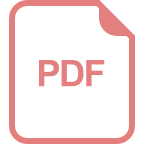
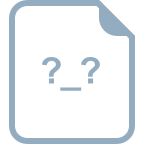


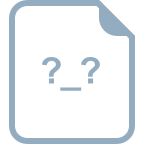
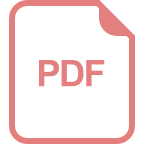
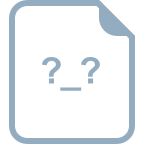
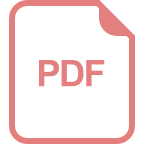
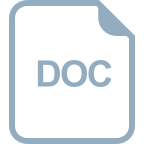
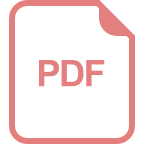
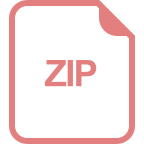
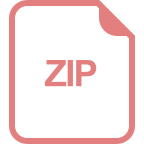