给我利用Python+OpenCV实现照片中的道路检测(lane detection)的代码
时间: 2023-06-04 14:03:50 浏览: 244
好的,以下是利用Python OpenCV实现照片中的道路检测(lane detection)的代码:
```
import cv2
import numpy as np
def region_of_interest(img, vertices):
mask = np.zeros_like(img)
# 填充三角形区域
cv2.fillPoly(mask, vertices, 255)
masked_img = cv2.bitwise_and(img, mask)
return masked_img
def draw_lines(img, lines, color=[0, 0, 255], thickness=3):
# 创建一个空的坐标列表,用于存放左、右车道线的所有坐标点
left_line = []
right_line = []
for line in lines:
x1, y1, x2, y2 = line.reshape(4)
# 计算线段斜率
slope = (y2 - y1) / (x2 - x1)
# 排除垂直线和水平线
if slope != np.nan and abs(slope) > 0.5:
# 分类左、右车道线的坐标点
if slope < 0:
left_line.append((x1, y1))
left_line.append((x2, y2))
else:
right_line.append((x1, y1))
right_line.append((x2, y2))
if len(left_line) > 0 and len(right_line) > 0:
# 将车道线的所有坐标点转换为 NumPy 数组
left_line = np.array(left_line)
right_line = np.array(right_line)
# 计算左、右车道线的一次多项式
left_fit = np.polyfit(left_line[:, 0], left_line[:, 1], 1)
right_fit = np.polyfit(right_line[:, 0], right_line[:, 1], 1)
# 计算左、右车道线与 ROI 底部的交点坐标
y1 = img.shape[0]
x1_l = int((y1 - left_fit[1]) / left_fit[0])
x1_r = int((y1 - right_fit[1]) / right_fit[0])
# 计算左、右车道线与 ROI 上部的交点坐标
y2 = int(img.shape[0] * 0.6)
x2_l = int((y2 - left_fit[1]) / left_fit[0])
x2_r = int((y2 - right_fit[1]) / right_fit[0])
# 画出左、右车道线
cv2.line(img, (x1_l, y1), (x2_l, y2), color, thickness)
cv2.line(img, (x1_r, y1), (x2_r, y2), color, thickness)
def process_image(img):
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行高斯滤波
kernel_size = 5
blur_gray = cv2.GaussianBlur(gray, (kernel_size, kernel_size), 0)
# 进行 Canny 边缘检测
low_threshold = 50
high_threshold = 150
edges = cv2.Canny(blur_gray, low_threshold, high_threshold)
# 设置 ROI 区域的顶点坐标
imshape = img.shape
vertices = np.array([[(100, imshape[0]), (450, 320), (520, 320), (imshape[1]-100, imshape[0])]], dtype=np.int32)
roi_image = region_of_interest(edges, vertices)
# 进行 Hough 变换检测直线
rho = 2
theta = np.pi/180
threshold = 100
min_line_len = 50
max_line_gap = 160
lines = cv2.HoughLinesP(roi_image, rho, theta, threshold, np.array([]), min_line_len, max_line_gap)
# 将检测到的直线画在原图上
line_image = np.zeros_like(img)
draw_lines(line_image, lines)
# 将检测到的车道线与原图进行叠加
result = cv2.addWeighted(img, 0.8, line_image, 1, 0)
return result
# 对输入视频进行车道线检测并显示处理结果
cap = cv2.VideoCapture('test_video.mp4')
while cap.isOpened():
ret, frame = cap.read()
if ret == True:
result = process_image(frame)
cv2.imshow('result', result)
if cv2.waitKey(25) & 0xFF == ord('q'):
break
else:
break
cap.release()
cv2.destroyAllWindows()
```
以上代码实现了利用 Python OpenCV 对照片中的道路进行检测的功能。其中,车道线检测算法使用了霍夫变换,可以检测出道路上的直线,然后通过一些数学方法来筛选出车道线的坐标点,并将它们转换为一次多项式。最终,我们可以将车道线与原图进行叠加,得到检测结果。
阅读全文
相关推荐
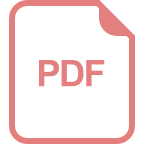
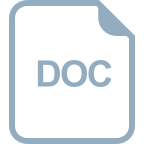
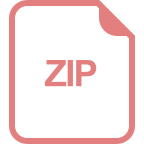
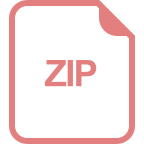
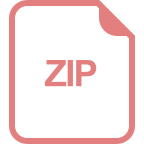
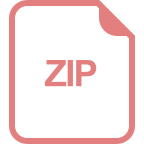
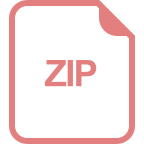
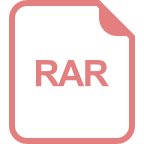
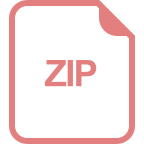
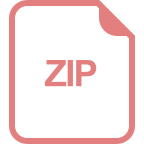
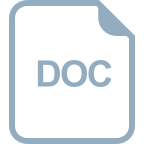
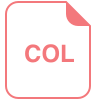




