用C语言实现:两个有序单链表的合并到新的链表
时间: 2024-02-09 17:10:35 浏览: 109
可以按照以下步骤来实现两个有序单链表的合并到新的链表:
1. 定义链表节点结构体,包含数据和指向下一个节点的指针。
```c
struct ListNode {
int val;
struct ListNode *next;
};
```
2. 实现一个函数 `mergeTwoLists`,接收两个链表的头指针,返回合并后的链表头指针。
```c
struct ListNode* mergeTwoLists(struct ListNode* l1, struct ListNode* l2) {
// 如果两个链表中有一个为空,则直接返回另一个链表
if (l1 == NULL) return l2;
if (l2 == NULL) return l1;
// 定义一个新链表的头指针和尾指针
struct ListNode dummy;
struct ListNode *tail = &dummy;
// 遍历两个链表,将较小值的节点插入到新链表的尾部
while (l1 != NULL && l2 != NULL) {
if (l1->val < l2->val) {
tail->next = l1;
l1 = l1->next;
} else {
tail->next = l2;
l2 = l2->next;
}
tail = tail->next;
}
// 将剩余的节点插入到新链表尾部
if (l1 != NULL) tail->next = l1;
if (l2 != NULL) tail->next = l2;
// 返回新链表的头指针
return dummy.next;
}
```
这个函数会遍历两个链表,将较小值的节点插入到新链表的尾部,最后返回新链表的头指针。
可以使用以下代码测试这个函数的正确性:
```c
int main() {
struct ListNode l1_3 = {4, NULL};
struct ListNode l1_2 = {2, &l1_3};
struct ListNode l1_1 = {1, &l1_2};
struct ListNode l2_3 = {7, NULL};
struct ListNode l2_2 = {3, &l2_3};
struct ListNode l2_1 = {1, &l2_2};
struct ListNode *merged = mergeTwoLists(&l1_1, &l2_1);
while (merged != NULL) {
printf("%d ", merged->val);
merged = merged->next;
}
printf("\n");
return 0;
}
```
输出应该为:`1 1 2 3 4 7`。
阅读全文
相关推荐
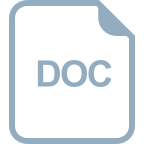
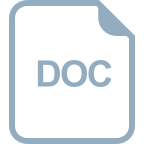
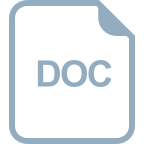







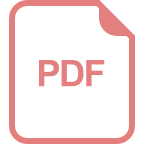
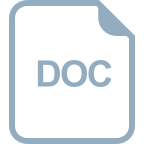






