使用pytroch的一维CNN模型预测土壤有机质含量
时间: 2024-03-05 11:54:59 浏览: 53
以下是使用PyTorch实现一维CNN模型预测土壤有机质含量的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# 加载数据
data = pd.read_csv('soil_data.csv')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
# 数据标准化
scaler = StandardScaler()
X = scaler.fit_transform(X)
# 数据划分
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 转换为Tensor
X_train = torch.from_numpy(X_train).float()
X_test = torch.from_numpy(X_test).float()
y_train = torch.from_numpy(y_train).float()
y_test = torch.from_numpy(y_test).float()
# 定义模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv1d(in_channels=1, out_channels=16, kernel_size=3)
self.pool = nn.MaxPool1d(kernel_size=2)
self.conv2 = nn.Conv1d(in_channels=16, out_channels=32, kernel_size=3)
self.fc1 = nn.Linear(in_features=32 * 23, out_features=64)
self.fc2 = nn.Linear(in_features=64, out_features=1)
def forward(self, x):
x = self.pool(torch.relu(self.conv1(x)))
x = self.pool(torch.relu(self.conv2(x)))
x = x.view(-1, 32 * 23)
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
net = Net()
# 定义损失函数和优化器
criterion = nn.MSELoss()
optimizer = optim.Adam(net.parameters(), lr=0.001)
# 训练模型
for epoch in range(100):
running_loss = 0.0
for i in range(len(X_train)):
optimizer.zero_grad()
outputs = net(X_train[i].unsqueeze(0).unsqueeze(0))
loss = criterion(outputs, y_train[i].unsqueeze(0))
loss.backward()
optimizer.step()
running_loss += loss.item()
print('Epoch %d loss: %.3f' % (epoch + 1, running_loss / len(X_train)))
# 测试模型
with torch.no_grad():
y_pred = net(X_test.unsqueeze(1))
mse = criterion(y_pred, y_test.unsqueeze(1))
print('MSE: %.3f' % mse)
```
在这个示例中,我们首先加载并标准化了土壤数据,然后将其分为训练集和测试集。接下来,我们定义了一个包含两个卷积层和两个全连接层的一维CNN模型,并使用均方误差作为损失函数和Adam优化器来训练模型。最后,我们使用训练好的模型在测试集上进行预测,并计算均方误差。
阅读全文
相关推荐
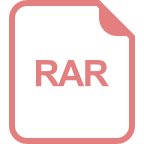
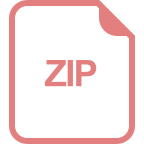
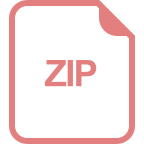
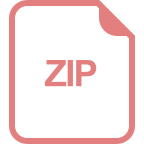
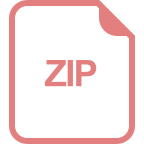
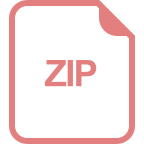
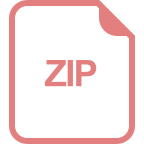
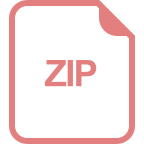
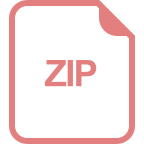
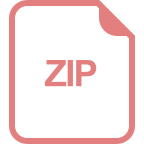
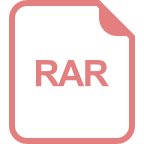
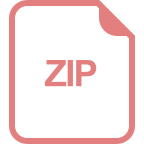
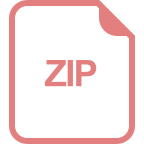