写一个数据库人事管理系统程序
时间: 2023-12-12 09:05:11 浏览: 32
这是一个比较大的项目,需要考虑到很多方面,包括数据结构设计、用户界面设计、安全性、性能等等。因此,我会给出一个大致的程序框架,供您参考。
1. 数据库设计
首先,需要设计数据库结构,包括员工表、部门表、职位表等等。以下是一个简单的员工表设计:
```
CREATE TABLE employee (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
gender ENUM('男', '女') NOT NULL,
birthday DATE NOT NULL,
department_id INT NOT NULL,
position_id INT NOT NULL,
salary FLOAT NOT NULL
);
```
2. 用户界面设计
接下来,需要设计用户界面,让用户可以方便地进行操作。可以使用 Java Swing 或者其他 GUI 工具包来实现。
3. 数据库连接
在程序中需要连接到数据库,可以使用 JDBC API 进行连接,以下是一个简单的连接示例:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class Database {
private static final String URL = "jdbc:mysql://localhost:3306/hr";
private static final String USER = "root";
private static final String PASSWORD = "123456";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
}
```
4. 功能实现
在程序中,需要实现一些基本的员工管理功能,如添加新员工、删除员工、更新员工信息、查询员工信息等等。以下是一个简单的添加员工信息的示例代码:
```java
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class EmployeeDAO {
private Connection connection;
public EmployeeDAO(Connection connection) {
this.connection = connection;
}
public void addEmployee(Employee employee) throws SQLException {
String sql = "INSERT INTO employee (name, gender, birthday, department_id, position_id, salary)"
+ " VALUES (?, ?, ?, ?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, employee.getName());
statement.setString(2, employee.getGender());
statement.setDate(3, employee.getBirthday());
statement.setInt(4, employee.getDepartmentId());
statement.setInt(5, employee.getPositionId());
statement.setFloat(6, employee.getSalary());
statement.executeUpdate();
}
}
```
5. 安全性
在程序中,需要注意安全性问题,如防止 SQL 注入攻击、保护数据库账号密码等等。可以使用 PreparedStatement 来预编译 SQL 语句,防止 SQL 注入攻击。同时,需要将数据库账号密码保存在配置文件中,而非直接写在代码中。
6. 性能
在程序中,需要考虑到性能问题,如如何优化查询语句、如何减少数据库连接次数等等。可以使用索引来优化查询语句,使用连接池来减少数据库连接次数。
以上是一个大致的数据库人事管理系统程序框架,仅供参考。具体实现需要根据具体需求进行调整。
相关推荐
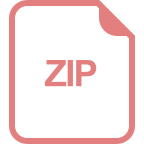














