已知数据集linearRegression_data.txt采用梯度下降的方法建立线性回归模型python代码怎么实现?
时间: 2024-03-25 12:39:58 浏览: 79
以下是使用梯度下降法实现线性回归模型的Python代码:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# 加载数据集
data = pd.read_csv('linearRegression_data.txt', header=None, names=['x', 'y'])
# 特征缩放
data['x'] = (data['x'] - data['x'].mean()) / data['x'].std()
# 添加一列全为1的向量作为截距
data.insert(0, 'Ones', 1)
# 将数据集分为特征和目标变量
X = np.matrix(data.iloc[:, :-1].values)
y = np.matrix(data.iloc[:, -1:].values)
# 初始化theta
theta = np.matrix(np.zeros((1, X.shape[1])))
# 定义代价函数
def computeCost(X, y, theta):
inner = np.power(((X * theta.T) - y), 2)
return np.sum(inner) / (2 * len(X))
# 定义梯度下降函数
def gradientDescent(X, y, theta, alpha, iters):
temp = np.matrix(np.zeros(theta.shape))
parameters = int(theta.ravel().shape[1])
cost = np.zeros(iters)
for i in range(iters):
error = (X * theta.T) - y
for j in range(parameters):
term = np.multiply(error, X[:, j])
temp[0, j] = theta[0, j] - ((alpha / len(X)) * np.sum(term))
theta = temp
cost[i] = computeCost(X, y, theta)
return theta, cost
# 设置学习率和迭代次数
alpha = 0.01
iters = 1000
# 运行梯度下降算法
theta, cost = gradientDescent(X, y, theta, alpha, iters)
# 输出最终的theta值
print(theta)
# 绘制代价函数随迭代次数的变化图
fig, ax = plt.subplots()
ax.plot(np.arange(iters), cost, 'r')
ax.set_xlabel('Iterations')
ax.set_ylabel('Cost')
ax.set_title('Error vs. Training Epoch')
plt.show()
```
上述代码中,我们首先加载数据集,并进行特征缩放,然后添加一列全为1的向量作为截距。接着,我们将数据集分为特征和目标变量,并初始化theta。然后,我们定义代价函数和梯度下降函数。在梯度下降函数中,我们迭代地更新theta,并计算代价函数的值。最后,我们设置学习率和迭代次数,并运行梯度下降算法。最终,输出得到的theta值,并绘制代价函数随迭代次数的变化图。
阅读全文
相关推荐
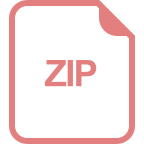

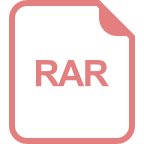
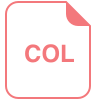
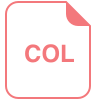
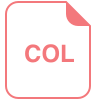
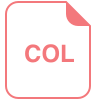
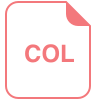
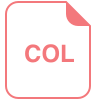
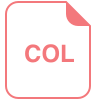
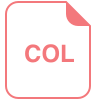
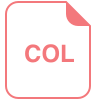
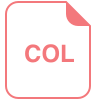
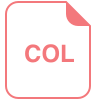
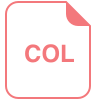
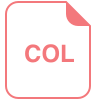
