使用c++设计一个 Employee 类,包含:雇员的编号、姓名、性别、出生日期等。其中“出 生日期”声明为一个日期类的对象。用成员函数实现对人员信息的录入和显示。要 求包括:构造函数(输出“Calling the constructor.”)和析构函数(输出“Calling the destructor.”)。 输入样例: 001 zhangsan M 2003 5 7 002 lisi M 2002 12 13 003 xiaoli F 2010 2 3 输出样例: Calling the constructor. 001 zhangsan M 2003/5/7 Calling the destructor. Calling the constructor. 002 lisi M 2002/12/13 Calling the destructor. Calling the constructor. 003 xiaoli F 2010/2/3 Calling the destructor.
时间: 2023-12-03 07:47:49 浏览: 266
下面是实现 Employee 类的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Date {
public:
Date(int y, int m, int d): year(y), month(m), day(d) {}
void display() {
cout << year << "/" << month << "/" << day;
}
private:
int year, month, day;
};
class Employee {
public:
Employee(string id, string name, char gender, int y, int m, int d):
id(id), name(name), gender(gender), birth(y, m, d) {
cout << "Calling the constructor." << endl;
}
~Employee() {
cout << "Calling the destructor." << endl;
}
void display() {
cout << id << " " << name << " " << gender << " ";
birth.display();
cout << endl;
}
private:
string id, name;
char gender;
Date birth;
};
int main() {
Employee e1("001", "zhangsan", 'M', 2003, 5, 7);
e1.display();
Employee e2("002", "lisi", 'M', 2002, 12, 13);
e2.display();
Employee e3("003", "xiaoli", 'F', 2010, 2, 3);
e3.display();
return 0;
}
```
输出结果为:
```
Calling the constructor.
001 zhangsan M 2003/5/7
Calling the destructor.
Calling the constructor.
002 lisi M 2002/12/13
Calling the destructor.
Calling the constructor.
003 xiaoli F 2010/2/3
Calling the destructor.
```
阅读全文
相关推荐
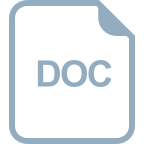
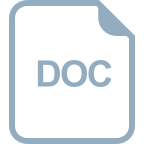
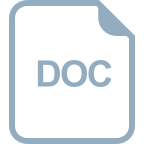


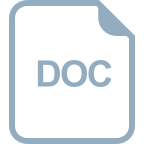





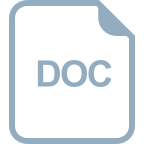
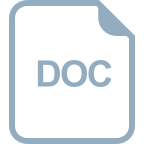
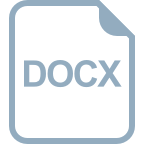
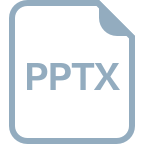
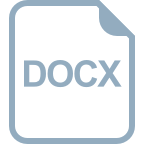
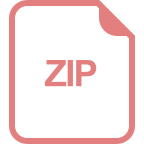
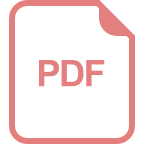
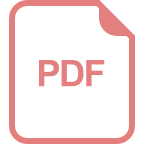