using c++ to implement a class of triangle
时间: 2024-05-26 10:10:00 浏览: 109
Sure, to implement a class of triangle in C, you can create a structure for the triangle with three variables for the three sides, and then define functions for computing the perimeter, area, and angles of the triangle. The functions can use the formulae for calculating these quantities based on the sides of the triangle.
相关问题
Using c++ to implement a class of Triangle.
Sure, I can help you with that! Creating a class for Triangle in C is fairly simple. You'll need to define the class with three properties for the three sides of the triangle and some methods to calculate its area and perimeter. Is there anything specific you need help with?
using c++ to implement a class of trian gle
Sure, to implement a class of triangle using C, you can define a structure with three variables representing the length of each side of the triangle. Then, you can write functions to compute the area and perimeter of the triangle based on these side lengths. Would you like me to provide sample code for this?
阅读全文
相关推荐
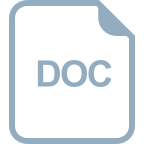
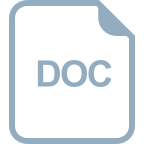
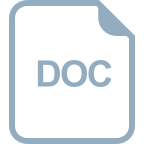
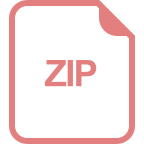
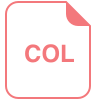
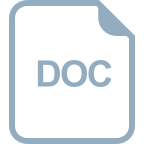
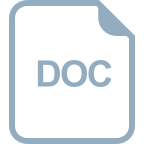
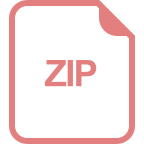
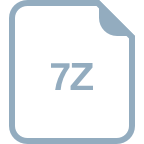
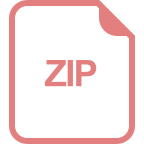