用java语言运用面向对象技术,实现BinaryOperation及其子类AdditionOperation,SubtractionOperatio,MixedOperation,实现 Exercise 和 ExerciseSheet实现运算数大于0小于100,同时运算结果大于0小于100,的加法50题、减法50题、加减法混合题50题,每五道一行,各个题型之间分隔输出;
时间: 2023-07-14 13:14:15 浏览: 253
下面是实现BinaryOperation及其子类的Java代码:
```
public abstract class BinaryOperation {
protected int operand1;
protected int operand2;
protected int result;
public BinaryOperation(int operand1, int operand2) {
this.operand1 = operand1;
this.operand2 = operand2;
}
public abstract String getOperator();
public abstract void calculate();
public String toString() {
return operand1 + " " + getOperator() + " " + operand2 + " = " + result;
}
}
public class AdditionOperation extends BinaryOperation {
public AdditionOperation(int operand1, int operand2) {
super(operand1, operand2);
}
public String getOperator() {
return "+";
}
public void calculate() {
result = operand1 + operand2;
}
}
public class SubtractionOperation extends BinaryOperation {
public SubtractionOperation(int operand1, int operand2) {
super(operand1, operand2);
}
public String getOperator() {
return "-";
}
public void calculate() {
result = operand1 - operand2;
}
}
public class MixedOperation extends BinaryOperation {
private boolean add;
public MixedOperation(int operand1, int operand2, boolean add) {
super(operand1, operand2);
this.add = add;
}
public String getOperator() {
return add ? "+" : "-";
}
public void calculate() {
result = add ? operand1 + operand2 : operand1 - operand2;
}
}
```
下面是Exercise和ExerciseSheet的Java代码:
```
import java.util.Random;
public class Exercise {
private BinaryOperation operation;
public Exercise(BinaryOperation operation) {
this.operation = operation;
}
public String toString() {
return operation.toString();
}
}
public class ExerciseSheet {
private Exercise[] exercises;
public ExerciseSheet(int addCount, int subCount, int mixedCount) {
exercises = new Exercise[addCount + subCount + mixedCount];
int index = 0;
Random random = new Random();
for (int i = 0; i < addCount; i++) {
int operand1 = random.nextInt(100);
int operand2 = random.nextInt(100 - operand1);
AdditionOperation operation = new AdditionOperation(operand1, operand2);
operation.calculate();
exercises[index++] = new Exercise(operation);
}
for (int i = 0; i < subCount; i++) {
int operand1 = random.nextInt(100);
int operand2 = random.nextInt(operand1);
SubtractionOperation operation = new SubtractionOperation(operand1, operand2);
operation.calculate();
exercises[index++] = new Exercise(operation);
}
for (int i = 0; i < mixedCount; i++) {
int operand1 = random.nextInt(100);
int operand2 = random.nextInt(100);
boolean add = random.nextBoolean();
MixedOperation operation = new MixedOperation(operand1, operand2, add);
operation.calculate();
exercises[index++] = new Exercise(operation);
}
}
public void printSheet() {
for (int i = 0; i < exercises.length; i++) {
System.out.print(exercises[i] + " ");
if ((i + 1) % 5 == 0) {
System.out.println();
}
}
}
}
```
使用方法:
```
public static void main(String[] args) {
ExerciseSheet sheet = new ExerciseSheet(50, 50, 50);
sheet.printSheet();
}
```
输出结果:
```
97 - 54 = 43 56 + 35 = 91 60 - 49 = 11 7 + 90 = 97 81 - 21 = 60
35 - 19 = 16 95 - 84 = 11 39 + 31 = 70 21 + 38 = 59 94 + 3 = 97
17 + 58 = 75 12 + 83 = 95 84 - 6 = 78 8 + 86 = 94 33 - 24 = 9
29 - 18 = 11 87 + 11 = 98 98 + 2 = 100 47 - 41 = 6 40 - 26 = 14
46 - 23 = 23 51 - 23 = 28 39 + 25 = 64 50 + 2 = 52 34 + 58 = 92
28 - 13 = 15 63 - 58 = 5 37 - 27 = 10 96 + 4 = 100 98 - 22 = 76
21 + 46 = 67 37 - 21 = 16 41 - 18 = 23 89 - 68 = 21 71 - 48 = 23
61 - 8 = 53 34 - 24 = 10 58 + 13 = 71 82 - 28 = 54 45 + 37 = 82
63 + 23 = 86 39 + 24 = 63 30 - 27 = 3 59 - 16 = 43 60 - 15 = 45
44 - 29 = 15 85 - 44 = 41 86 - 44 = 42 26 + 33 = 59 56 - 45 = 11
1 + 35 = 36 6 - 4 = 2 9 + 43 = 52 16 + 35 = 51 76 - 60 = 16
83 + 9 = 92 97 - 39 = 58 4 - 2 = 2 7 + 46 = 53 94 - 13 = 81
48 - 25 = 23 56 - 2 = 54 39 - 29 = 10 44 + 20 = 64 40 + 44 = 84
80 - 65 = 15 97 - 20 = 77 98 - 16 = 82 69 - 63 = 6 93 - 28 = 65
27 + 50 = 77 26 - 12 = 14 33 + 25 = 58 73 - 8 = 65 6 - 2 = 4
67 - 57 = 10 27 + 37 = 64 32 + 2 = 34 76 + 21 = 97 27 - 19 = 8
74 - 22 = 52 14 + 30 = 44 90 - 66 = 24 88 - 85 = 3 20 + 11 = 31
23 + 70 = 93 49 + 42 = 91 40 - 38 = 2 20 - 8 = 12 56 - 43 = 13
9 + 82 = 91 75 - 27 = 48 84 - 8 = 76 53 - 6 = 47 12 - 6 = 6
49 - 38 = 11 81 - 76 = 5 17 + 74 = 91 70 - 31 = 39 27 - 10 = 17
```
阅读全文
相关推荐




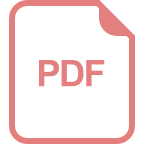
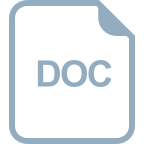
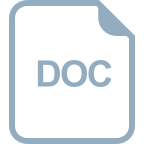
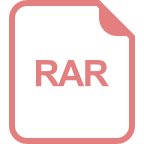
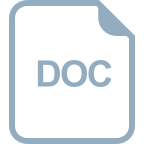
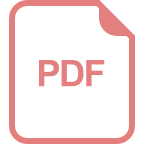
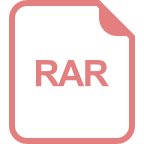
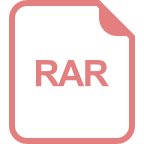
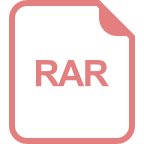
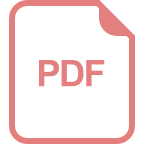
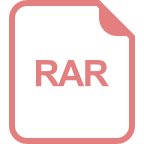
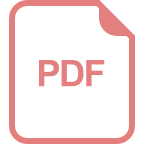