用Python从http://zhushou.360.cn/list/index/批量下载apk到本地
时间: 2023-07-11 11:28:11 浏览: 251
你可以使用 Python 的 requests 库和 BeautifulSoup 库来批量下载 http://zhushou.360.cn/list/index/ 上的 APK 文件。以下是一个示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 待下载的应用分类列表
categories = ['hot', 'new', 'reco', 'sort']
# 遍历应用分类列表
for category in categories:
# 构造分类页面 URL
url = f'http://zhushou.360.cn/list/index/cid/{category}/page/1'
# 发送 GET 请求并获取响应
response = requests.get(url)
# 解析响应内容
soup = BeautifulSoup(response.content, 'html.parser')
# 查找所有应用的下载链接
app_links = soup.select('.SeaCon .SeaList li a.btn-install')
# 遍历应用下载链接列表
for app_link in app_links:
# 获取 APK 下载链接和文件名
download_url = app_link['href']
filename = download_url.split('/')[-1]
# 发送 GET 请求并获取响应
response = requests.get(download_url)
# 检查响应状态码是否为 200
if response.status_code == 200:
# 将响应内容写入文件
with open(filename, 'wb') as f:
f.write(response.content)
print(f'APK 文件已成功下载到本地:{filename}')
else:
print(f'下载失败:{download_url}')
```
在这个示例中,我们首先定义了一个应用分类列表,然后遍历该列表并构造分类页面的 URL。然后,我们使用 `requests.get()` 方法发送 GET 请求并获取响应内容,将其传递给 BeautifulSoup 对象进行解析。我们使用 CSS 选择器 `.SeaCon .SeaList li a.btn-install` 查找所有应用的下载链接,然后遍历下载链接列表,从链接中获取 APK 文件的下载链接和文件名,并使用 `requests.get()` 方法发送 GET 请求并将响应内容写入文件。最后,我们输出成功下载的文件名。如果下载失败,则输出错误信息。
阅读全文
相关推荐
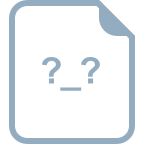
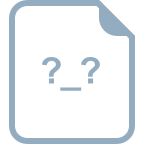
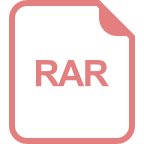
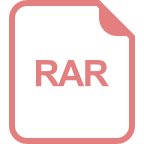
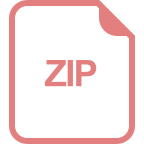
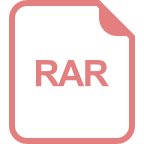
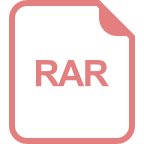
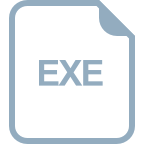
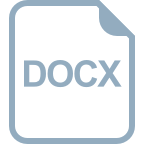
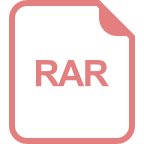
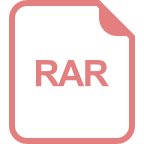
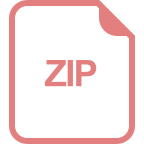
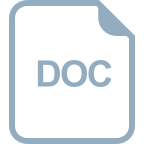
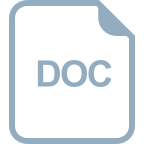