(1)用C语言编程,打开/etc/passwd文件,找出已经注册的普通用户账号 (2)用C语言实现多进程编程,主进程每隔1秒获取当前系统时间写入某文件,子进程打开该文件,读取文件的内容并显示在屏幕上。
时间: 2023-06-16 08:05:10 浏览: 56
1. 用C语言编程,打开/etc/passwd文件,找出已经注册的普通用户账号
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <pwd.h>
int main() {
FILE *fp;
char line[100];
struct passwd *pw;
if ((fp = fopen("/etc/passwd", "r")) == NULL) {
perror("fopen");
exit(1);
}
while (fgets(line, sizeof(line), fp) != NULL) {
pw = getpwent();
if (pw != NULL && pw->pw_uid >= 1000 && pw->pw_uid < 65534) {
printf("%s\n", pw->pw_name);
}
}
fclose(fp);
return 0;
}
```
2. 用C语言实现多进程编程,主进程每隔1秒获取当前系统时间写入某文件,子进程打开该文件,读取文件的内容并显示在屏幕上。
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
#include <time.h>
void child_process(char* filename) {
FILE* fp;
char buf[100];
while (1) {
fp = fopen(filename, "r");
if (fp == NULL) {
perror("fopen");
exit(1);
}
fgets(buf, sizeof(buf), fp);
printf("%s", buf);
fclose(fp);
sleep(1);
}
}
int main() {
pid_t pid;
char* filename = "time.txt";
time_t now;
struct tm* tm_info;
FILE* fp;
fp = fopen(filename, "w");
if (fp == NULL) {
perror("fopen");
exit(1);
}
pid = fork();
if (pid == -1) {
perror("fork");
exit(1);
} else if (pid == 0) {
child_process(filename);
} else {
while (1) {
time(&now);
tm_info = localtime(&now);
fprintf(fp, "%d:%d:%d\n", tm_info->tm_hour, tm_info->tm_min, tm_info->tm_sec);
fflush(fp);
sleep(1);
wait(NULL);
}
fclose(fp);
}
return 0;
}
```
相关推荐
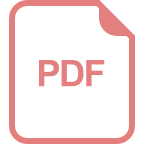














