C++ 使用正则表达式 封装解析json工具类
时间: 2023-10-27 15:13:26 浏览: 164

c++用的正则表达式包

在C++中,使用正则表达式封装解析JSON工具类可以方便地解析JSON格式的字符串。下面是一个简单的例子:
```cpp
#include <iostream>
#include <regex>
#include <string>
#include <vector>
#include <map>
class JsonParser {
public:
JsonParser() {}
std::map<std::string, std::string> parse(std::string jsonStr) {
std::map<std::string, std::string> jsonMap;
std::regex reg("\"(.*?)\":\"(.*?)\""); // 匹配 key:value 的正则表达式
std::sregex_iterator it(jsonStr.begin(), jsonStr.end(), reg);
std::sregex_iterator end;
while (it != end) {
std::smatch match = *it;
jsonMap[match.str(1)] = match.str(2);
++it;
}
return jsonMap;
}
};
int main() {
std::string jsonStr = "{\"name\":\"Tom\",\"age\":\"20\",\"address\":\"Shanghai\"}";
JsonParser parser;
std::map<std::string, std::string> jsonMap = parser.parse(jsonStr);
for (auto &p : jsonMap) {
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
```
在上面的代码中,我们定义了一个`JsonParser`类,并实现了`parse`方法来解析JSON格式的字符串。在`parse`方法中,我们使用了正则表达式来匹配JSON字符串中的`key:value`键值对,并将其存入一个`map`中。最后,我们可以遍历这个`map`来输出解析结果。
需要注意的是,这里只是一个简单的例子,实际的JSON解析工具类可能需要处理更复杂的JSON格式,例如嵌套的JSON对象或数组。因此,我们需要更复杂的正则表达式来匹配这些格式,并编写更复杂的代码来解析它们。
阅读全文
相关推荐
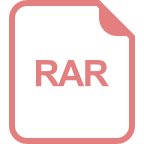
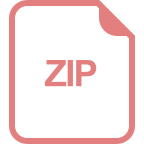
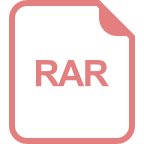
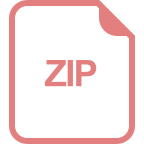
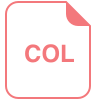
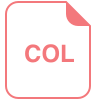
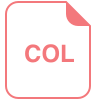
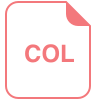
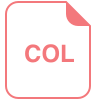
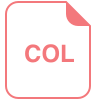
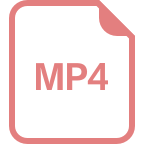
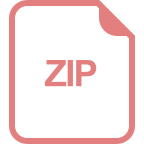
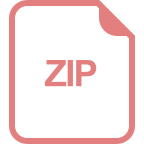