写一段cuda计算sha256的代码
时间: 2023-09-12 07:05:58 浏览: 112
### 回答1:
以下是一段用 CUDA 计算 SHA256 的代码:
```
#include <iostream>
#include <cuda.h>
#include <openssl/sha.h>
__global__ void sha256(const char *input, char *output, size_t length) {
SHA256_CTX ctx;
SHA256_Init(&ctx);
SHA256_Update(&ctx, input, length);
SHA256_Final((unsigned char *)output, &ctx);
}
int main() {
const char *input = "hello world";
char output[SHA256_DIGEST_LENGTH];
size_t length = strlen(input);
char *d_input, *d_output;
cudaMalloc((void **)&d_input, length + 1);
cudaMalloc((void **)&d_output, SHA256_DIGEST_LENGTH);
cudaMemcpy(d_input, input, length + 1, cudaMemcpyHostToDevice);
sha256<<<1, 1>>>(d_input, d_output, length);
cudaMemcpy(output, d_output, SHA256_DIGEST_LENGTH, cudaMemcpyDeviceToHost);
for (int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
printf("%02x", (unsigned int)output[i]);
}
printf("\n");
cudaFree(d_input);
cudaFree(d_output);
return 0;
}
```
请注意,在使用此代码前,您需要安装 CUDA 和 OpenSSL,并在您的编译器中链接它们。
### 回答2:
以下是一个使用CUDA计算SHA256散列的示例代码:
```cpp
#include <stdio.h>
#include <stdint.h>
#include <cuda_runtime.h>
#include <cuda.h>
#include <cuda_runtime_api.h>
#include <openssl/sha.h>
__global__
void sha256Hash(uint8_t *data, uint8_t *hash) {
int idx = threadIdx.x;
unsigned char temp[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
SHA256_Update(&sha256, data, blockDim.x);
SHA256_Final(temp, &sha256);
for (int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
hash[idx * SHA256_DIGEST_LENGTH + i] = temp[i];
}
}
int main() {
uint8_t inputData[1024]; // 输入数据
uint8_t hash[1024]; // 计算出的SHA256散列
cudaError_t cudaStatus;
uint8_t *dev_inputData;
uint8_t *dev_hash;
// 在GPU上分配内存
cudaStatus = cudaMalloc((void**)&dev_inputData, 1024);
if (cudaStatus != cudaSuccess) {
fprintf(stderr, "cudaMalloc failed!");
return 1;
}
cudaStatus = cudaMalloc((void**)&dev_hash, 1024);
if (cudaStatus != cudaSuccess) {
fprintf(stderr, "cudaMalloc failed!");
cudaFree(dev_inputData);
return 1;
}
// 复制输入数据到GPU
cudaStatus = cudaMemcpy(dev_inputData, inputData, 1024, cudaMemcpyHostToDevice);
if (cudaStatus != cudaSuccess) {
fprintf(stderr, "cudaMemcpy failed!");
cudaFree(dev_inputData);
cudaFree(dev_hash);
return 1;
}
// 调用CUDA核函数计算SHA256散列
sha256Hash<<<1, 1024>>>(dev_inputData, dev_hash);
// 复制计算结果从GPU到主机内存
cudaStatus = cudaMemcpy(hash, dev_hash, 1024, cudaMemcpyDeviceToHost);
if (cudaStatus != cudaSuccess) {
fprintf(stderr, "cudaMemcpy failed!");
cudaFree(dev_inputData);
cudaFree(dev_hash);
return 1;
}
// 打印计算结果
for (int i = 0; i < 1024; i += SHA256_DIGEST_LENGTH) {
printf("Hash %d: ", i / SHA256_DIGEST_LENGTH);
for (int j = 0; j < SHA256_DIGEST_LENGTH; j++) {
printf("%02x ", hash[i + j]);
}
printf("\n");
}
// 释放GPU内存
cudaFree(dev_inputData);
cudaFree(dev_hash);
return 0;
}
```
该代码使用OpenSSL库中的SHA256哈希函数。首先,需要在计算密集型的`sha256Hash`函数上声明一个CUDA核函数,并使用CUDA设备内存进行输入数据和散列结果的存储。
在主函数中,我们首先在GPU上分配内存,然后将输入数据从主机复制到设备。接着,我们通过调用CUDA核函数来计算SHA256散列。最后,我们将计算结果从设备复制回主机以进行打印和后续处理。最后,我们释放GPU上的内存。
请注意,该示例代码仅供参考,并且可能需要进一步完善以适应特定的应用场景和数据处理需求。
### 回答3:
CUDA(Compute Unified Device Architecture)是NVIDIA开发的一种并行计算平台和编程模型。它可以利用GPU(Graphics Processing Unit)进行高性能的并行计算。
下面是一段使用CUDA来计算SHA256的伪代码:
```c++
#include <iostream>
#include <sstream>
#include <iomanip>
#include <cuda.h>
#include <openssl/sha.h>
// 定义CUDA核函数
__global__ void sha256_cuda(unsigned char* input, unsigned char* output) {
int tid = threadIdx.x + blockIdx.x * blockDim.x;
// 使用CUDA的SHA256库计算哈希值
SHA256_CTX ctx;
SHA256_Init(&ctx);
SHA256_Update(&ctx, input, sizeof(input));
SHA256_Final(output, &ctx);
}
int main() {
// 准备输入数据
unsigned char input[] = "Hello, world!";
unsigned char* d_input;
unsigned char* d_output;
// 在GPU上分配内存
cudaMalloc(&d_input, sizeof(input));
cudaMalloc(&d_output, SHA256_DIGEST_LENGTH);
// 将输入数据从主机内存拷贝到设备内存
cudaMemcpy(d_input, input, sizeof(input), cudaMemcpyHostToDevice);
// 调用CUDA核函数计算SHA256
sha256_cuda<<<1, 1>>>(d_input, d_output);
// 从设备内存拷贝结果到主机内存
unsigned char output[SHA256_DIGEST_LENGTH];
cudaMemcpy(output, d_output, SHA256_DIGEST_LENGTH, cudaMemcpyDeviceToHost);
// 打印SHA256哈希值
std::ostringstream oss;
for (int i = 0; i < SHA256_DIGEST_LENGTH; ++i) {
oss << std::hex << std::setw(2) << std::setfill('0') << static_cast<int>(output[i]);
}
std::cout << "SHA256: " << oss.str() << std::endl;
// 释放GPU内存
cudaFree(d_input);
cudaFree(d_output);
return 0;
}
```
上述代码使用CUDA在GPU上计算SHA256哈希值。首先,通过`cudaMalloc`函数在GPU上分配内存来存储输入数据和输出结果。然后,使用`cudaMemcpy`函数将输入数据从主机内存拷贝到设备内存。接下来,调用CUDA核函数`sha256_cuda`,并指定需要启动的线程块和每个线程块中的线程数。在核函数内部,使用CUDA的SHA256库计算哈希值,并将结果保存在设备内存中。最后,使用`cudaMemcpy`函数将结果从设备内存拷贝到主机内存,并打印出SHA256哈希值。
请注意,上述代码是伪代码,具体实现可能会因为CUDA版本、编译器和硬件设备的差异而有所变化。实际使用时需要根据具体情况进行适当的修改和调整。
相关推荐
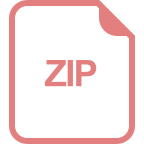
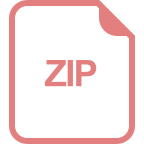














