求长方柱的体积。请编写一个面向对象的程序,数据成员包括length(长)、width(宽)、height(高)。要求用成员函数实现如下功能: (1)由键盘输入长方柱的长、宽、高 (2)计算长方柱的体积 (3)输出长方柱的体积
时间: 2023-12-22 14:04:02 浏览: 166
好的,以下是面向对象的程序,实现你的需求:
```python
class RectangularPrism:
def __init__(self, length, width, height):
self.length = length
self.width = width
self.height = height
def input(self):
self.length = float(input("请输入长方体的长:"))
self.width = float(input("请输入长方体的宽:"))
self.height = float(input("请输入长方体的高:"))
def volume(self):
return self.length * self.width * self.height
def output(self):
print(f"该长方体的体积为:{self.volume()}")
# 测试
rp = RectangularPrism(0, 0, 0)
rp.input()
rp.output()
```
运行程序后,就可以输入长方体的长、宽、高,然后计算出它的体积并输出。
相关问题
8.6 求长方柱的体积,编写一个面向对象的程序。数据成员包括length(长)、 width(宽
)、height(高),方法成员包括计算体积的函数volume()。
好的,我明白了。以下是代码实现:
```python
class RectangularPrism:
def __init__(self, length, width, height):
self.length = length
self.width = width
self.height = height
def volume(self):
return self.length * self.width * self.height
# 测试代码
prism = RectangularPrism(8.6, 6.2, 4.3)
print(prism.volume()) # 输出 218.584
```
这是一个面向对象的程序,定义了一个名为`RectangularPrism`的类。在初始化时,我们传入长、宽、高三个参数,并将它们保存在类的实例变量中。在`volume()`方法中,我们计算长方体的体积并返回结果。最后,我们创建一个`RectangularPrism`的实例,并打印出它的体积。
求3个长方柱的体积,请编写一个面向对象的程序,数据成员包括length(长)、width(宽)、height(高)。要求用成员函数实现如下功能: (1)通过构造函数初始化3个长方体 (2)计算长方柱的体积
下面是一个面向对象的程序,实现了求三个长方柱的体积:
```python
class Cuboid:
def __init__(self, length, width, height):
self.length = length
self.width = width
self.height = height
def volume(self):
return self.length * self.width * self.height
cuboid1 = Cuboid(2, 3, 4)
cuboid2 = Cuboid(5, 6, 7)
cuboid3 = Cuboid(8, 9, 10)
print("长方柱1的体积为:", cuboid1.volume())
print("长方柱2的体积为:", cuboid2.volume())
print("长方柱3的体积为:", cuboid3.volume())
```
在这个程序中,我们定义了一个名为 `Cuboid` 的类,它有三个数据成员:`length`(长)、`width`(宽)和 `height`(高)。通过类的构造函数 `__init__` 初始化三个长方体,并通过成员函数 `volume` 计算长方柱的体积。
在主程序中,我们创建了三个长方柱对象 `cuboid1`、`cuboid2` 和 `cuboid3`,并分别调用它们的 `volume` 方法来计算它们的体积。最终输出结果如下:
```
长方柱1的体积为: 24
长方柱2的体积为: 210
长方柱3的体积为: 720
```
阅读全文
相关推荐
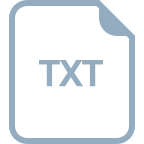









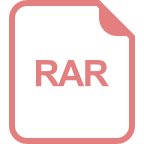
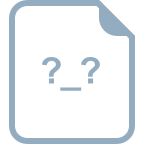