A*算法实现,python代码
时间: 2023-09-03 08:28:03 浏览: 111
### 回答1:
好的,这是一个 A* 算法的 Python 实现代码示例:
```python
import heapq
import math
class Node:
def __init__(self, x, y, cost, priority):
self.x = x
self.y = y
self.cost = cost
self.priority = priority
def __lt__(self, other):
return self.priority < other.priority
def astar(maze, start, end):
start_node = Node(start[0], start[1], 0, 0)
end_node = Node(end[0], end[1], 0, 0)
heap = []
heapq.heappush(heap, start_node)
came_from = {}
cost_so_far = {}
came_from[start_node] = None
cost_so_far[start_node] = 0
while heap:
current = heapq.heappop(heap)
if current.x == end_node.x and current.y == end_node.y:
break
neighbors = [(current.x + dx, current.y + dy) for dx, dy in [(1, 0), (-1, 0), (0, 1), (0, -1)]]
for next in neighbors:
x, y = next
if 0 <= x < len(maze) and 0 <= y < len(maze[0]) and maze[x][y] != "#":
new_cost = cost_so_far[current] + 1
next_node = Node(x, y, new_cost, 0)
if next_node not in cost_so_far or new_cost < cost_so_far[next_node]:
cost_so_far[next_node] = new_cost
priority = new_cost + math.sqrt((end_node.x - x) ** 2 + (end_node.y - y) ** 2)
next_node.priority = priority
heapq.heappush(heap, next_node)
came_from[next_node] = current
return came_from, cost_so_far
```
上面的代码是 A* 算法的核心实现,其中 `maze` 是迷宫地图,`start` 是起点坐标,`end` 是终点坐标。它返回两个字典 `came_from` 和 `cost_so_far`,分别表示从起点到每个点的最短路径和最小代价。
### 回答2:
A*算法是一种常用于寻路问题的启发式搜索算法,下面是一个使用Python实现的简单示例代码:
```python
from queue import PriorityQueue
# 创建优先级队列用于存储待探索的节点
open_list = PriorityQueue()
# 创建字典用于存储已探索过的节点
closed_list = {}
# 创建字典用于存储每个节点的父节点
parents = {}
# 创建字典用于存储每个节点的估计路径成本
g_scores = {}
# 创建字典用于存储每个节点的启发式估计值
h_scores = {}
# 定义启发式估计函数,这里使用曼哈顿距离作为启发式估计值
def heuristic(current, goal):
return abs(current[0] - goal[0]) + abs(current[1] - goal[1])
# 定义A*算法函数
def A_star_search(start, goal, graph):
# 将起始节点加入优先级队列,并设置起始节点的路径成本为0
open_list.put((0, start))
g_scores[start] = 0
while not open_list.empty():
# 从优先级队列中取出路径成本最低的节点
current = open_list.get()[1]
if current == goal:
path = []
# 根据父节点关系回溯找到最终路径
while current in parents:
path.append(current)
current = parents[current]
return path[::-1]
# 将当前节点加入已探索节点字典
closed_list[current] = True
# 遍历当前节点的邻居节点
for neighbor in graph[current]:
# 计算邻居节点的路径成本
g_score = g_scores[current] + graph[current][neighbor]
if neighbor not in g_scores or g_score < g_scores[neighbor]:
# 更新邻居节点的路径成本和启发式估计值
g_scores[neighbor] = g_score
h_score = heuristic(neighbor, goal)
f_score = g_score + h_score
# 更新邻居节点的父节点
parents[neighbor] = current
# 将邻居节点加入优先级队列
open_list.put((f_score, neighbor))
return None
# 定义图的邻接表表示
graph = {
'A': {'B': 5, 'C': 3},
'B': {'D': 2},
'C': {'D': 6, 'E': 8},
'D': {'E': 7, 'F': 9},
'E': {'F': 2},
'F': {}
}
start_node = 'A'
goal_node = 'F'
# 执行A*算法并打印结果
path = A_star_search(start_node, goal_node, graph)
print("最短路径:", path)
```
以上是一个简单的使用Python实现的A*算法示例代码,通过定义启发式估计函数、优先级队列等数据结构和算法逻辑,可以有效地寻找起始节点到目标节点的最短路径。
### 回答3:
A*算法是一种常用的启发式搜索算法,用于求解图中的最短路径问题。以下是一个用Python实现的A*算法代码示例:
```
# 估算从当前节点到目标节点的代价
def heuristic_cost_estimate(current, goal):
return abs(current[0] - goal[0]) + abs(current[1] - goal[1])
# 获取当前节点的邻居节点
def get_neighbors(node, grid):
neighbors = []
directions = [(1, 0), (-1, 0), (0, 1), (0, -1)] # 右、左、下、上
for direction in directions:
neighbor = (node[0] + direction[0], node[1] + direction[1])
if 0 <= neighbor[0] < len(grid) and 0 <= neighbor[1] < len(grid[0]) and grid[neighbor[0]][neighbor[1]] == 0:
neighbors.append(neighbor)
return neighbors
# 执行A*算法
def astar(start, goal, grid):
open_set = [start]
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic_cost_estimate(start, goal)}
while open_set:
current = min(open_set, key=lambda x: f_score[x])
if current == goal:
# 返回最短路径
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
open_set.remove(current)
neighbors = get_neighbors(current, grid)
for neighbor in neighbors:
tentative_g_score = g_score[current] + 1 # 相邻节点距离为1
if neighbor not in g_score or tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = g_score[neighbor] + heuristic_cost_estimate(neighbor, goal)
if neighbor not in open_set:
open_set.append(neighbor)
return None
```
上述代码中,`heuristic_cost_estimate`函数用于估算从当前节点到目标节点的代价。`get_neighbors`函数用于获取当前节点的邻居节点,其中`grid`表示节点的二维矩阵,`0`表示可行走的节点。
`astar`函数执行A*算法,其中`start`表示起始节点,`goal`表示目标节点,`grid`表示节点的二维矩阵。算法使用了开放集合`open_set`、来自字典`came_from`、实际代价字典`g_score`和估计代价字典`f_score`来辅助搜索。算法通过循环遍历open_set中的节点,计算最短路径,并返回最短路径。
该代码示例可用于求解智能体在栅格地图中从起点到终点的最短路径问题。
阅读全文
相关推荐














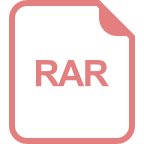

