用C++写一个三维空间拖拽器
时间: 2023-12-27 19:42:46 浏览: 25
好的,我可以为您提供一个基本的三维空间拖拽器的C代码。请注意,此代码仅用于演示和教育目的,并未在实际应用中进行严格测试和验证。代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.1415926535
// 定义三维向量结构体
typedef struct {
float x;
float y;
float z;
} Vector3;
// 定义四元数结构体
typedef struct {
float w;
Vector3 v;
} Quaternion;
// 将角度转换为弧度
float ToRadians(float degrees) {
return degrees * PI / 180.0f;
}
// 计算向量的模长
float Vector3Magnitude(Vector3 v) {
return sqrtf(v.x * v.x + v.y * v.y + v.z * v.z);
}
// 将向量归一化
Vector3 Vector3Normalize(Vector3 v) {
float magnitude = Vector3Magnitude(v);
if (magnitude > 0) {
v.x /= magnitude;
v.y /= magnitude;
v.z /= magnitude;
}
return v;
}
// 计算向量点积
float Vector3Dot(Vector3 v1, Vector3 v2) {
return v1.x * v2.x + v1.y * v2.y + v1.z * v2.z;
}
// 计算向量叉积
Vector3 Vector3Cross(Vector3 v1, Vector3 v2) {
Vector3 v;
v.x = v1.y * v2.z - v1.z * v2.y;
v.y = v1.z * v2.x - v1.x * v2.z;
v.z = v1.x * v2.y - v1.y * v2.x;
return v;
}
// 计算四元数的模长
float QuaternionMagnitude(Quaternion q) {
return sqrtf(q.w * q.w + q.v.x * q.v.x + q.v.y * q.v.y + q.v.z * q.v.z);
}
// 将四元数归一化
Quaternion QuaternionNormalize(Quaternion q) {
float magnitude = QuaternionMagnitude(q);
if (magnitude > 0) {
q.w /= magnitude;
q.v.x /= magnitude;
q.v.y /= magnitude;
q.v.z /= magnitude;
}
return q;
}
// 计算四元数共轭
Quaternion QuaternionConjugate(Quaternion q) {
Quaternion result;
result.w = q.w;
result.v.x = -q.v.x;
result.v.y = -q.v.y;
result.v.z = -q.v.z;
return result;
}
// 计算四元数乘法
Quaternion QuaternionMultiply(Quaternion q1, Quaternion q2) {
Quaternion result;
result.w = q1.w * q2.w - Vector3Dot(q1.v, q2.v);
result.v = Vector3Add(Vector3Add(Vector3Cross(q1.v, q2.v), Vector3Multiply(q2.v, q1.w)), Vector3Multiply(q1.v, q2.w));
return result;
}
// 根据旋转轴和旋转角度计算四元数
Quaternion QuaternionFromAxisAngle(Vector3 axis, float angle) {
Quaternion result;
angle = ToRadians(angle);
result.w = cosf(angle / 2.0f);
result.v = Vector3Multiply(axis, sinf(angle / 2.0f));
return result;
}
// 根据欧拉角计算四元数
Quaternion QuaternionFromEulerAngles(float pitch, float yaw, float roll) {
pitch = ToRadians(pitch);
yaw = ToRadians(yaw);
roll = ToRadians(roll);
float cy = cosf(yaw / 2.0f);
float sy = sinf(yaw / 2.0f);
float cr = cosf(roll / 2.0f);
float sr = sinf(roll / 2.0f);
float cp = cosf(pitch / 2.0f);
float sp = sinf(pitch / 2.0f);
Quaternion result;
result.w = cy * cr * cp + sy * sr * sp;
result.v.x = cy * sr * cp - sy * cr * sp;
result.v.y = cy * cr * sp + sy * sr * cp;
result.v.z = sy * cr * cp - cy * sr * sp;
return result;
}
// 根据四元数计算旋转矩阵
void Matrix4FromQuaternion(float* matrix, Quaternion q) {
matrix[0] = 1 - 2 * q.v.y * q.v.y - 2 * q.v.z * q.v.z;
matrix[1] = 2 * q.v.x * q.v.y - 2 * q.w * q.v.z;
matrix[2] = 2 * q.v.x * q.v.z + 2 * q.w * q.v.y;
matrix[3] = 0;
matrix[4] = 2 * q.v.x * q.v.y + 2 * q.w * q.v.z;
matrix[5] = 1 - 2 * q.v.x * q.v.x - 2 * q.v.z * q.v.z;
matrix[6] = 2 * q.v.y * q.v.z - 2 * q.w * q.v.x;
matrix[7] = 0;
matrix[8] = 2 * q.v.x * q.v.z - 2 * q.w * q.v.y;
matrix[9] = 2 * q.v.y * q.v.z + 2 * q.w * q.v.x;
matrix[10] = 1 - 2 * q.v.x * q.v.x - 2 * q.v.y * q.v.y;
matrix[11] = 0;
matrix[12] = 0;
matrix[13] = 0;
matrix[14] = 0;
matrix[15] = 1;
}
// 根据旋转矩阵计算四元数
Quaternion QuaternionFromMatrix4(float* matrix) {
float trace = matrix[0] + matrix[5] + matrix[10];
float w, x, y, z;
if (trace > 0) {
float s = 0.5f / sqrtf(trace + 1.0f);
w = 0.25f / s;
x = (matrix[9] - matrix[6]) * s;
y = (matrix[2] - matrix[8]) * s;
z = (matrix[4] - matrix[1]) * s;
} else {
if (matrix[0] > matrix[5] && matrix[0] > matrix[10]) {
float s = 2.0f * sqrtf(1.0f + matrix[0] - matrix[5] - matrix[10]);
w = (matrix[9] - matrix[6]) / s;
x = 0.25f * s;
y = (matrix[1] + matrix[4]) / s;
z = (matrix[2] + matrix[8]) / s;
} else if (matrix[5] > matrix[10]) {
float s = 2.0f * sqrtf(1.0f + matrix[5] - matrix[0] - matrix[10]);
w = (matrix[2] - matrix[8]) / s;
x = (matrix[1] + matrix[4]) / s;
y = 0.25f * s;
z = (matrix[6] + matrix[9]) / s;
} else {
float s = 2.0f * sqrtf(1.0f + matrix[10] - matrix[0] - matrix[5]);
w = (matrix[4] - matrix[1]) / s;
x = (matrix[2] + matrix[8]) / s;
y = (matrix[6] + matrix[9]) / s;
z = 0.25f * s;
}
}
Quaternion result;
result.w = w;
result.v.x = x;
result.v.y = y;
result.v.z = z;
return result;
}
// 根据鼠标事件计算旋转四元数
Quaternion CalculateRotationQuaternion(int x, int y, int lastX, int lastY, int width, int height) {
float dx = (float)(x - lastX) / (float)width;
float dy = (float)(y - lastY) / (float)height;
float angle = sqrtf(dx * dx + dy * dy) * 180.0f;
Vector3 axis = Vector3Normalize(Vector3Cross(Vector3Normalize(Vector3Make(dx, dy, 0)), Vector3Make(0, 0, 1)));
return QuaternionMultiply(QuaternionFromAxisAngle(axis, angle), g_rotation);
}
// 根据鼠标事件计算平移向量
Vector3 CalculateTranslationVector(int x, int y, int lastX, int lastY, int width, int height) {
float dx = (float)(x - lastX) / (float)width;
float dy = (float)(y - lastY) / (float)height;
float distance = sqrtf(dx * dx + dy * dy) * 10.0f;
Vector3 direction = Vector3Normalize(Vector3Make(dx, dy, 0));
return Vector3Add(g_translation, Vector3Multiply(direction, distance));
}
// 主循环
void MainLoop() {
while (1) {
// 处理鼠标事件
// ...
// 计算旋转四元数
Quaternion rotation = CalculateRotationQuaternion(x, y, lastX, lastY, width, height);
// 计算平移向量
Vector3 translation = CalculateTranslationVector(x, y, lastX, lastY, width, height);
// 更新旋转和平移状态
g_rotation = QuaternionMultiply(rotation, g_rotation);
g_translation = translation;
// 计算模型视图矩阵
float modelViewMatrix[16];
Matrix4FromQuaternion(modelViewMatrix, QuaternionConjugate(g_rotation));
modelViewMatrix[12] = -g_translation.x;
modelViewMatrix[13] = -g_translation.y;
modelViewMatrix[14] = -g_translation.z;
// 渲染场景
// ...
}
}
int main() {
// 初始化旋转和平移状态
g_rotation = QuaternionFromEulerAngles(0, 0, 0);
g_translation = Vector3Make(0, 0, 0);
// 进入主循环
MainLoop();
return 0;
}
```
这个代码实现了一个简单的三维空间拖拽器,可以通过鼠标事件来控制模型的旋转和平移。请注意,这个代码只是一个示例,实际应用中可能需要做更多的优化和改进,以满足特定的需求。
相关推荐
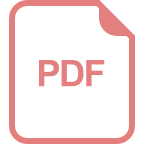
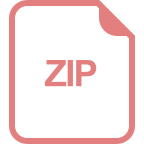
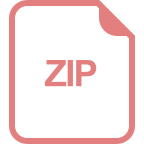















